OOP (C++) Tutorial
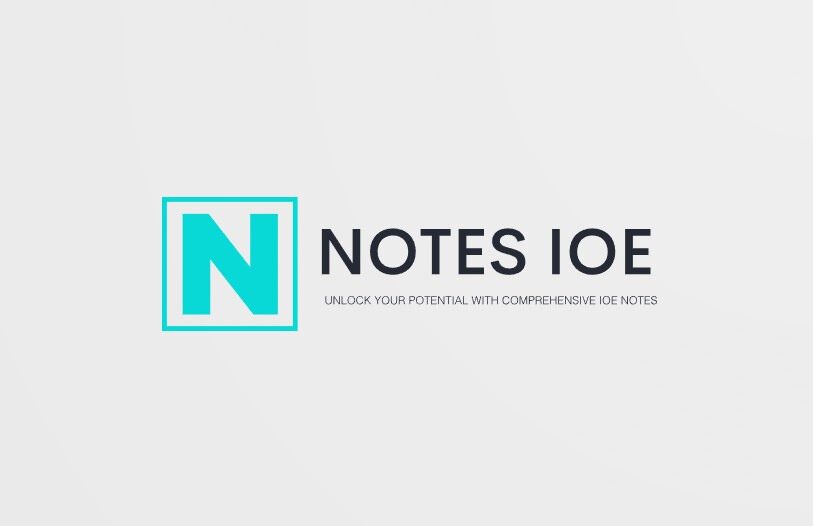
Part 1
1. Write a program to calculate and display the cube of integer, float and double
numbers using function overloading (passing a single argument to a function).
Source Code:
#include <iostream>
#include <cmath>
using namespace std;
class overload{
public:
// Function to calculate the cube of a int
int cube(int num) {
return pow(num,3);
}
// Function to calculate the cube of a float
float cube(float num) {
return pow(num,3);
}
// Function to calculate the cube of a double
double cube(double num) {
return pow(num,3);
}
};
int main(){
overload s;
int integer_number = 5;
float float_number = 2.5;
double double_number = 1.75;
cout<<"Cube of "<<integer_number<< " is: "<<s.cube(integer_number)<<endl;
cout<<"Cube of "<<float_number<< " is: "<<s.cube(float_number)<<endl;
cout<<"Cube of "<<double_number<<" is: "<<s.cube(double_number)<<endl;
return 0;
}
Output:
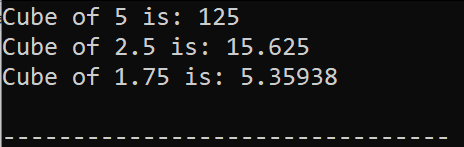
2. Write a program to find the smallest and largest number from an array using DMA with new and delete operators.
Source Code:
#include <iostream>
using namespace std;
class sl{
int i,j,x,n,*p;
public:
void num_count(){
cout<<"How manu number you want to enter? ";
cin>>n;
}
void large_small(){
p=new int [n];
for(i=0;i<n;i++){
cout<<"\nEnter "<<i+1<<" Number: ";
cin>>p[i];
}
for(i=0;i<n;i++){
for(j=i+1;j<n;j++){
if(p[i]>p[j]){
x=p[i];
p[i]=p[j];
p[j]=x;
}
}
}
}
void display(){
cout<<"The largest number is: "<<p[n-1];
cout<<"\nThe smallest number is: "<<p[0];
delete []p;
}
};
int main(){
sl s;
s.num_count();
s.large_small();
s.display();
}
Output:
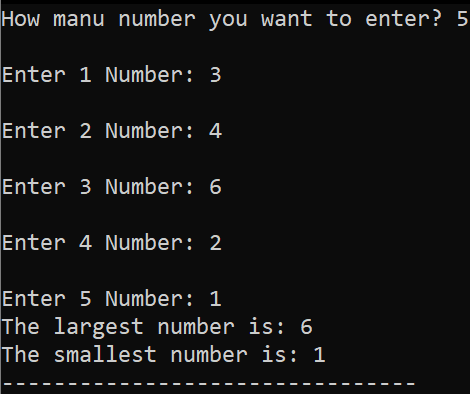
3. Write a function to pass two objects of type LandMeasure and return their sum. (16 Ana = 1 Ropani, 4 Paisa= 1 Ana, 4 Dam= 1 Paisa
Source Code:
#include <iostream>
class LandMeasure {
private:
int ropani;
int ana;
int paisa;
int dam;
public:
LandMeasure(int r, int a, int p, int d) : ropani(r), ana(a), paisa(p), dam(d) {}
int getRopani() const {
return ropani;
}
int getAna() const {
return ana;
}
int getPaisa() const {
return paisa;
}
int getDam() const {
return dam;
}
};
LandMeasure addLandMeasures(const LandMeasure& measure1, const LandMeasure& measure2) {
int totalRopani = measure1.getRopani() + measure2.getRopani();
int totalAna = measure1.getAna() + measure2.getAna();
int totalPaisa = measure1.getPaisa() + measure2.getPaisa();
int totalDam = measure1.getDam() + measure2.getDam();
// Convert to higher units
totalAna += totalPaisa / 4;
totalPaisa %= 4;
totalRopani += totalAna / 16;
totalAna %= 16;
return LandMeasure(totalRopani, totalAna, totalPaisa, totalDam);
}
int main() {
LandMeasure measure1(2, 10, 2, 1); // Example: 2 Ropani 10 Ana 2 Paisa 1 Dam
LandMeasure measure2(1, 7, 3, 3); // Example: 1 Ropani 7 Ana 3 Paisa 3 Dam
LandMeasure totalMeasure = addLandMeasures(measure1, measure2);
std::cout << "Total Land Measure: "
<< totalMeasure.getRopani() << " Ropani "
<< totalMeasure.getAna() << " Ana "
<< totalMeasure.getPaisa() << " Paisa "
<< totalMeasure.getDam() << " Dam" << std::endl;
return 0;
}
In this example, the LandMeasure
class represents the land measurement with different units (Ropani, Ana, Paisa, and Dam). The addLandMeasures
function takes two LandMeasure
objects and calculates their sum, properly converting units to higher levels as needed. The main
function demonstrates how to use the function with example land measurements.
Output:

4. Write a program that uses pass by reference to change meter to centimeter using pass by reference along with the namespace
Source Code:
#include <iostream>
namespace Conversion {
void metersToCentimeters(double& meters) {
meters *= 100;
}
}
int main() {
using namespace std;
double lengthInMeters;
cout << "Enter length in meters: ";
cin >> lengthInMeters;
Conversion::metersToCentimeters(lengthInMeters);
cout << "Length in centimeters: " << lengthInMeters << " cm" << endl;
return 0;
}
In this program, the Conversion
the namespace contains a function metersToCentimeters
that takes a double reference as a parameter. The function multiplies the value by 100 to convert it from meters to centimeters.
The main
the function reads the length in meters from the user, calls the conversion function using the namespace, and then displays the result in centimeters.
Output:
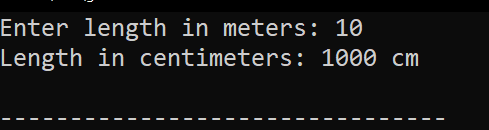
7. Create a class called carport that has int member data for car id, int member data for charge/hour, and float member data for time. Set the data and show the charges and parked hours of the corresponding car id. Make two members for setting and showing the data. Member functions should be called from other functions.
Source Code:
#include <iostream>
using namespace std;
class Carport {
private:
int car_id;
int charge_per_hour;
float parked_time;
public:
void set_data(int id, int charge, float time) {
car_id = id;
charge_per_hour = charge;
parked_time = time;
}
void show_data() {
float charges = charge_per_hour * parked_time;
cout << "Car ID: " << car_id <<endl;
cout << "Charge per hour: $" << charge_per_hour <<endl;
cout << "Parked hours: " << parked_time << " hours" <<endl;
cout << "\nTotal charges: $" << charges <<endl;
}
};
int main() {
Carport car1;
car1.set_data(1, 10, 2.5);
car1.show_data();
cout<<endl;
Carport car2;
car2.set_data(2, 15, 1.75);
car2.show_data();
return 0;
}
Output:
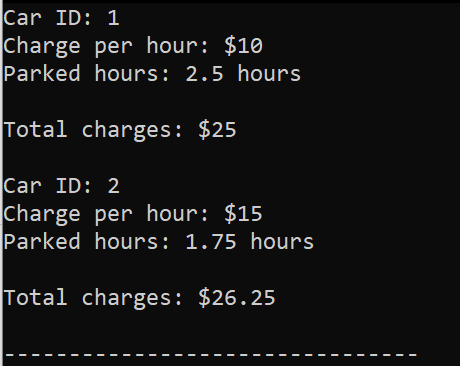
Part 2
8. Write a program to overload += operator. (You can overload this operator using
Distance class as d1 += d2)
Source Code
#include <iostream>
using namespace std;
class Distance {
private:
float feet;
float inches;
public:
Distance() : feet(0), inches(0) {}
Distance(float ft, float in) : feet(ft), inches(in) {}
void showDistance() const {
cout << feet << " feet " << inches << " inches" << endl;
}
// Overloading += operator
Distance& operator+=(const Distance& other) {
feet += other.feet;
inches += other.inches;
if (inches >= 12.0) {
feet += static_cast<int>(inches / 12);
inches = inches - static_cast<int>(inches / 12) * 12;
}
return *this;
}
};
int main() {
Distance d1(5, 10);
Distance d2(3, 8);
cout << "Distance 1: ";
d1.showDistance();
cout << "Distance 2: ";
d2.showDistance();
d1 += d2; // Using overloaded += operator
cout << "After adding Distance 2 to Distance 1: ";
d1.showDistance();
return 0;
}
Output:

11. Write a program to overload + operator to concatenate two strings.
Source Code:
#include <iostream>
#include <string.h>
using namespace std;
class stringcat {
char a[25];
public:
void stringinput() {
cout << "Enter string: ";
cin >> a;
}
void display() {
cout << a;
}
friend stringcat operator+(stringcat, stringcat);
};
stringcat operator+(stringcat A, stringcat B) {
stringcat C;
strcpy(C.a, A.a);
strcat(C.a, B.a);
return C;
}
int main() {
stringcat A, B, C;
A.stringinput();
B.stringinput();
C = A + B;
cout << "Concatenated string: ";
C.display();
return 0;
}
Output:
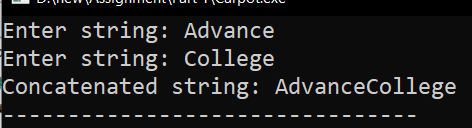
13. Develop a program using a class to with 3×3 matrix as a data menber. Overload the * operators so as to multiply two matrices.
Source Code:
#include <iostream>
using namespace std;
class Matrix3x3 {
private:
int data[3][3];
public:
Matrix3x3() {
// Initialize matrix with zeros
for (int i = 0; i < 3; ++i)
for (int j = 0; j < 3; ++j)
data[i][j] = 0;
}
Matrix3x3(int arr[3][3]) {
// Initialize matrix with provided array
for (int i = 0; i < 3; ++i)
for (int j = 0; j < 3; ++j)
data[i][j] = arr[i][j];
}
void display() const {
for (int i = 0; i < 3; ++i) {
for (int j = 0; j < 3; ++j)
cout << data[i][j] << "\t";
cout << endl;
}
}
Matrix3x3 operator*(const Matrix3x3& other) const {
Matrix3x3 result;
for (int i = 0; i < 3; ++i) {
for (int j = 0; j < 3; ++j) {
result.data[i][j] = 0;
for (int k = 0; k < 3; ++k) {
result.data[i][j] += data[i][k] * other.data[k][j];
}
}
}
return result;
}
};
int main() {
int arr1[3][3] = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
int arr2[3][3] = {{9, 8, 7}, {6, 5, 4}, {3, 2, 1}};
Matrix3x3 matrix1(arr1);
Matrix3x3 matrix2(arr2);
cout << "Matrix 1:" << endl;
matrix1.display();
cout << endl;
cout << "Matrix 2:" << endl;
matrix2.display();
cout << endl;
Matrix3x3 result = matrix1 * matrix2;
cout << "Matrix 1 * Matrix 2:" << endl;
result.display();
return 0;
}
Output:
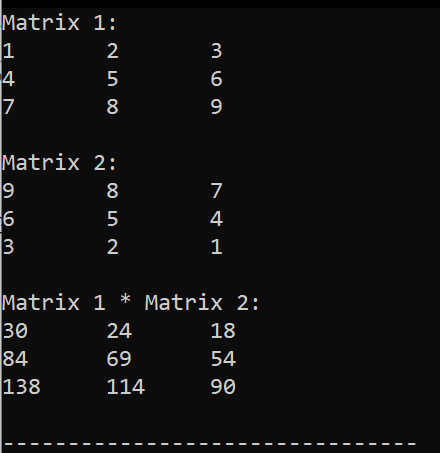
15. Create a class having an array as a member. Overload index operator ([ ]) to input and display the elements in the array.
Source Code:
#include <iostream>
class ArrayWithIndex {
private:
int size;
int* arr;
public:
ArrayWithIndex(int n) : size(n) {
arr = new int[size];
}
~ArrayWithIndex() {
delete[] arr;
}
int& operator[](int index) {
if (index < 0 || index >= size) {
std::cerr << "Index out of bounds!" << std::endl;
exit(1); // Terminate the program
}
return arr[index];
}
void display() const {
for (int i = 0; i < size; ++i) {
std::cout << arr[i] << " ";
}
std::cout << std::endl;
}
};
int main() {
int size;
std::cout << "Enter the size of the array: ";
std::cin >> size;
ArrayWithIndex arr(size);
std::cout << "Enter " << size << " elements:" << std::endl;
for (int i = 0; i < size; ++i) {
std::cin >> arr[i];
}
std::cout << "Entered elements: ";
arr.display();
return 0;
}
In this example, the ArrayWithIndex
class has an array as a member. The operator[]
is overloaded to provide access to array elements with proper bounds checking. The display()
function is used to display the elements in the array. The main
function demonstrates how to create an object of the class, input elements using the overloaded index operator, and then display the entered elements.
Output:
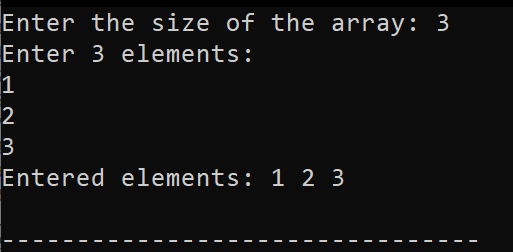
Part 3
19. Imagine a college hires some lecturers. Some lecturers are paid in period basis, while others are paid in month basis. Create a class called lecturer that stores the ID, and the name of lecturers. From this class derive two classes: PartTime, which adds payperhr (type float); and FullTime, which adds paypermonth (type float). Each of these three classes should have a readdata() function to get its data from the user, and a printdata() function to display its data. Write a main() program to test the FullTime and PartTime classes by creating instances of them, asking the user to fill in their data with readdata(), and then displaying the data with printdata().
Source Code
#include <iostream>
#include <string>
using namespace std;
class Lecturer {
protected:
int ID;
string name;
public:
void readdata() {
cout << "Enter ID: ";
cin >> ID;
cout << "Enter name: ";
cin.ignore(); // Clear newline character from previous input
getline(cin, name);
}
void printdata() {
cout << "ID: " << ID << endl;
cout << "Name: " << name << endl;
}
};
class PartTime : public Lecturer {
private:
float payperhr;
public:
void readdata() {
Lecturer::readdata();
cout << "Enter pay per hour: ";
cin >> payperhr;
}
void printdata() {
Lecturer::printdata();
cout << "Pay per hour: " << payperhr << endl;
}
};
class FullTime : public Lecturer {
private:
float paypermonth;
public:
void readdata() {
Lecturer::readdata();
cout << "Enter pay per month: ";
cin >> paypermonth;
}
void printdata() {
Lecturer::printdata();
cout << "Pay per month: " << paypermonth << endl;
}
};
int main() {
PartTime partTimeLecturer;
FullTime fullTimeLecturer;
cout << "Enter data for Part-Time Lecturer:" << endl;
partTimeLecturer.readdata();
cout << "Enter data for Full-Time Lecturer:" << endl;
fullTimeLecturer.readdata();
cout << "\nPart-Time Lecturer's Data:" << endl;
partTimeLecturer.printdata();
cout << "\nFull-Time Lecturer's Data:" << endl;
fullTimeLecturer.printdata();
return 0;
}
Output:
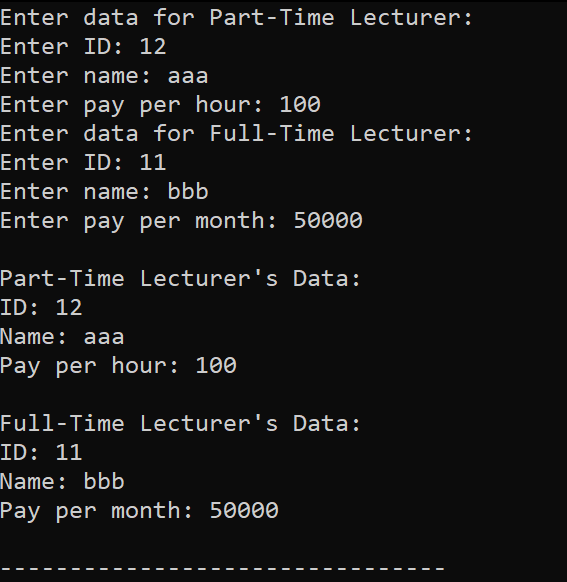
20. Create a class called cricketer with member variables to represent name, age and no of matches played. From this class derive two classes: Bowler and Batsman.
Bowler class has no_of_wickets as member variable and Batsman class has no_of_runs and centuries as member variables. Use appropriate member functions in all classes to read and display respective data.
Source Code:
#include <iostream>
#include <string>
using namespace std;
class Cricketer {
protected:
string name;
int age;
int matchesPlayed;
public:
void readData() {
cout << "Enter name: ";
cin.ignore(); // Clear newline character from previous input
getline(cin, name);
cout << "Enter age: ";
cin >> age;
cout << "Enter number of matches played: ";
cin >> matchesPlayed;
}
void displayData() {
cout << "Name: " << name << endl;
cout << "Age: " << age << endl;
cout << "Matches Played: " << matchesPlayed << endl;
}
};
class Bowler : public Cricketer {
private:
int no_of_wickets;
public:
void readData() {
Cricketer::readData();
cout << "Enter number of wickets: ";
cin >> no_of_wickets;
}
void displayData() {
Cricketer::displayData();
cout << "Number of wickets: " << no_of_wickets << endl;
}
};
class Batsman : public Cricketer {
private:
int no_of_runs;
int centuries;
public:
void readData() {
Cricketer::readData();
cout << "Enter number of runs: ";
cin >> no_of_runs;
cout << "Enter number of centuries: ";
cin >> centuries;
}
void displayData() {
Cricketer::displayData();
cout << "Number of runs: " << no_of_runs << endl;
cout << "Number of centuries: " << centuries << endl;
}
};
int main() {
Bowler bowler;
Batsman batsman;
cout << "Enter data for Bowler:" << endl;
bowler.readData();
cout << "Enter data for Batsman:" << endl;
batsman.readData();
cout << "\nBowler's Data:" << endl;
bowler.displayData();
cout << "\nBatsman's Data:" << endl;
batsman.displayData();
return 0;
}
Output
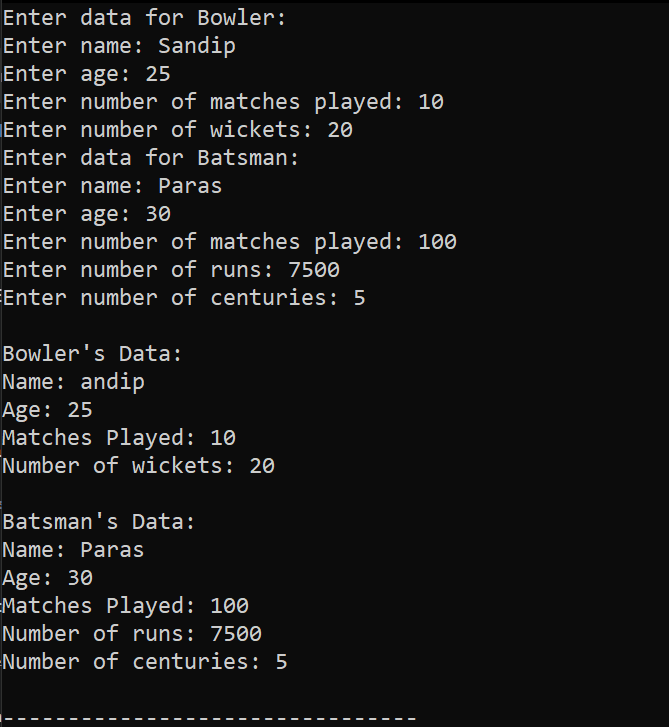
22. Create classes Book having data members name of author (string), price (float)
and class Stock having data members number of books (int) and category (string).
Create another class Library which derives from both the classes Book and
Stock. All the classes should have functions having same name. Write program to
test these classes.
Source Code:
#include <iostream>
#include <string>
class Book {
protected:
std::string author;
float price;
public:
Book(const std::string& a, float p) : author(a), price(p) {}
void display() {
std::cout << "Author: " << author << ", Price: $" << price << std::endl;
}
};
class Stock {
protected:
int numBooks;
std::string category;
public:
Stock(int n, const std::string& c) : numBooks(n), category(c) {}
void display() {
std::cout << "Number of books: " << numBooks << ", Category: " << category << std::endl;
}
};
class Library : public Book, public Stock {
public:
Library(const std::string& a, float p, int n, const std::string& c)
: Book(a, p), Stock(n, c) {}
void display() {
std::cout << "Library Information:" << std::endl;
Book::display();
Stock::display();
}
};
int main() {
Library library("John Doe", 25.99, 100, "Fiction");
library.display();
return 0;
}
In this program, the Book
class represents book information, the Stock
class represents stock information, and the Library
class is derived from both Book
and Stock
. The Library
class inherits the display()
functions from both base classes and provides its own version of display()
that calls the base class versions as well. The main
function creates an instance of the Library
class and tests the inheritance and polymorphism by calling the display()
function.
Output:
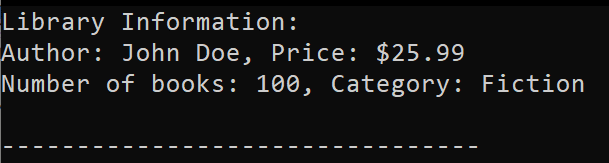
23. Create a class Person, create 2 derived classes Employee and Student inherited from class Person. Now create a class Manager which is derived from 2 base classes Employee and Student. Show the use of virtual class, virtual function, virtual destructor, order of invocation of constructor.
Source Code
#include <iostream>
#include <string>
using namespace std;
class Person {
protected:
string name;
public:
Person(const string& n) : name(n) {
cout << "Person constructor" << endl;
}
virtual void displayInfo() const {
cout << "Person Name: " << name << endl;
}
virtual ~Person() {
cout << "Person destructor" << endl;
}
};
class Employee : virtual public Person {
protected:
int employeeID;
public:
Employee(const string& n, int id) : Person(n), employeeID(id) {
cout << "Employee constructor" << endl;
}
virtual void displayInfo() const override {
Person::displayInfo();
cout << "Employee ID: " << employeeID << endl;
}
virtual ~Employee() {
cout << "Employee destructor" << endl;
}
};
class Student : virtual public Person {
protected:
int studentID;
public:
Student(const string& n, int id) : Person(n), studentID(id) {
cout << "Student constructor" << endl;
}
virtual void displayInfo() const override {
Person::displayInfo();
cout << "Student ID: " << studentID << endl;
}
virtual ~Student() {
cout << "Student destructor" << endl;
}
};
class Manager : public Employee, public Student {
protected:
int managerID;
public:
Manager(const string& n, int empID, int stuID, int mgrID)
: Person(n), Employee(n, empID), Student(n, stuID), managerID(mgrID) {
cout << "Manager constructor" << endl;
}
void displayInfo() const override {
Employee::displayInfo();
Student::displayInfo();
cout << "Manager ID: " << managerID << endl;
}
~Manager() {
cout << "Manager destructor" << endl;
}
};
int main() {
Manager manager("John", 101, 201, 301);
cout << "\nDisplaying Information:" << endl;
manager.displayInfo();
return 0;
}
In this example:
Person
,Employee
, andStudent
classes are created.Employee
andStudent
classes inherit virtually fromPerson
to avoid the diamond problem.virtual
is used to declare virtual functions to enable dynamic dispatch.- The
Manager
class inherits from bothEmployee
andStudent
. - Constructors and destructors are called in the order of inheritance during object creation and destruction.
Output
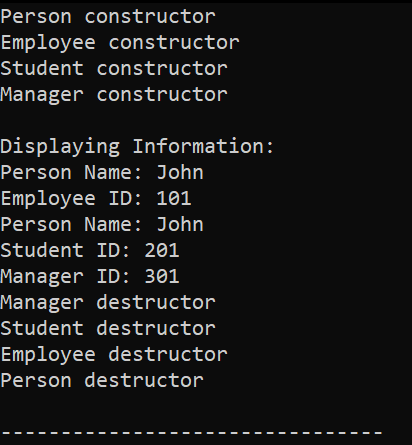
Part 4
27. Write a program for transaction processing that write and read random object to and from a random access file so that the user can add, update, delete and display the account information (account number, last name, first name, total balance).
Source Code
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
class Account {
public:
int accountNumber;
string lastName;
string firstName;
double totalBalance;
Account() : accountNumber(0), lastName(""), firstName(""), totalBalance(0.0) {}
Account(int accNum, const string& lName, const string& fName, double balance)
: accountNumber(accNum), lastName(lName), firstName(fName), totalBalance(balance) {}
};
void writeAccountToFile(const Account& account, int recordNumber) {
ofstream outFile("accounts.dat", ios::binary | ios::in | ios::out);
if (!outFile) {
cout << "Error opening file for writing!" << endl;
return;
}
outFile.seekp(recordNumber * sizeof(Account));
outFile.write(reinterpret_cast<const char*>(&account), sizeof(Account));
}
void readAccountFromFile(Account& account, int recordNumber) {
ifstream inFile("accounts.dat", ios::binary);
if (!inFile) {
cout << "Error opening file for reading!" << endl;
return;
}
inFile.seekg(recordNumber * sizeof(Account));
inFile.read(reinterpret_cast<char*>(&account), sizeof(Account));
}
void displayAccount(const Account& account) {
cout << "Account Number: " << account.accountNumber << endl;
cout << "Last Name: " << account.lastName << endl;
cout << "First Name: " << account.firstName << endl;
cout << "Total Balance: " << account.totalBalance << endl;
cout << "----------------------------------" << endl;
}
int main() {
int choice;
int accountNumber;
Account account;
do {
cout << "1. Add Account" << endl;
cout << "2. Update Account" << endl;
cout << "3. Delete Account" << endl;
cout << "4. Display Account" << endl;
cout << "5. Exit" << endl;
cout << "Enter your choice: ";
cin >> choice;
switch (choice) {
case 1: {
cout << "Enter Account Number: ";
cin >> account.accountNumber;
cout << "Enter Last Name: ";
cin >> account.lastName;
cout << "Enter First Name: ";
cin >> account.firstName;
cout << "Enter Total Balance: ";
cin >> account.totalBalance;
writeAccountToFile(account, account.accountNumber);
break;
}
case 2: {
cout << "Enter Account Number to Update: ";
cin >> accountNumber;
readAccountFromFile(account, accountNumber);
if (account.accountNumber != 0) {
cout << "Enter New Total Balance: ";
cin >> account.totalBalance;
writeAccountToFile(account, accountNumber);
} else {
cout << "Account not found!" << endl;
}
break;
}
case 3: {
cout << "Enter Account Number to Delete: ";
cin >> accountNumber;
Account emptyAccount;
writeAccountToFile(emptyAccount, accountNumber);
cout << "Account deleted." << endl;
break;
}
case 4: {
cout << "Enter Account Number to Display: ";
cin >> accountNumber;
readAccountFromFile(account, accountNumber);
if (account.accountNumber != 0) {
displayAccount(account);
} else {
cout << "Account not found!" << endl;
}
break;
}
case 5:
cout << "Exiting program." << endl;
break;
default:
cout << "Invalid choice. Please try again." << endl;
}
} while (choice != 5);
return 0;
}
Output:
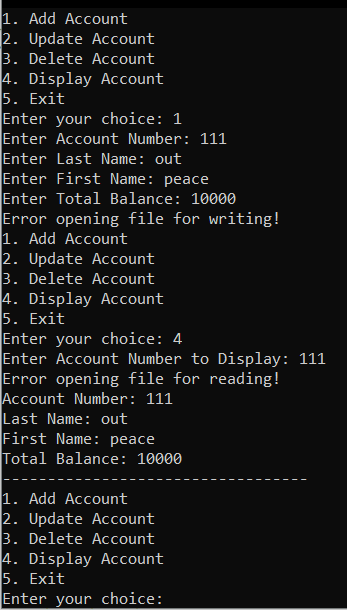
28. Write a program to make billing system of a department store. Your program should store and retrieve data to/from files. Use manipulators to display the record in proper formats.
Source Code
#include <iostream>
#include <fstream>
#include <iomanip>
#include <vector>
#include <string>
using namespace std;
class Item {
public:
string name;
double price;
int quantity;
Item(const string& n, double p, int q) : name(n), price(p), quantity(q) {}
};
class BillingSystem {
private:
vector<Item> items;
public:
void addItem(const string& name, double price, int quantity) {
items.push_back(Item(name, price, quantity));
}
void displayBill() {
double totalAmount = 0.0;
cout << "-----------------------------------------" << endl;
cout << setw(20) << left << "Item Name" << setw(10) << "Quantity" << setw(15) << "Price" << setw(15) << "Total" << endl;
cout << "-----------------------------------------" << endl;
for (vector<Item>::iterator it = items.begin(); it != items.end(); ++it) {
const Item& item = *it;
double totalItemAmount = item.price * item.quantity;
totalAmount += totalItemAmount;
cout << setw(20) << left << item.name
<< setw(10) << item.quantity
<< setw(15) << fixed << setprecision(2) << item.price
<< setw(15) << fixed << setprecision(2) << totalItemAmount << endl;
}
cout << "-----------------------------------------" << endl;
cout << setw(45) << "Total Amount: " << setw(15) << fixed << setprecision(2) << totalAmount << endl;
}
void saveToFile(const string& filename) {
ofstream outFile(filename.c_str()); // C++98 doesn't support passing strings directly
if (!outFile) {
cout << "Error opening file for writing!" << endl;
return;
}
for (vector<Item>::iterator it = items.begin(); it != items.end(); ++it) {
const Item& item = *it;
outFile << item.name << " " << item.price << " " << item.quantity << endl;
}
outFile.close();
}
void loadFromFile(const string& filename) {
ifstream inFile(filename.c_str()); // C++98 doesn't support passing strings directly
if (!inFile) {
cout << "Error opening file for reading!" << endl;
return;
}
items.clear();
string name;
double price;
int quantity;
while (inFile >> name >> price >> quantity) {
items.push_back(Item(name, price, quantity));
}
inFile.close();
}
};
int main() {
BillingSystem billingSystem;
billingSystem.loadFromFile("items.txt");
int choice;
do {
cout << "1. Add Item" << endl;
cout << "2. Display Bill" << endl;
cout << "3. Exit" << endl;
cout << "Enter your choice: ";
cin >> choice;
switch (choice) {
case 1: {
string name;
double price;
int quantity;
cout << "Enter item name: ";
cin.ignore();
getline(cin, name);
cout << "Enter item price: ";
cin >> price;
cout << "Enter item quantity: ";
cin >> quantity;
billingSystem.addItem(name, price, quantity);
break;
}
case 2:
billingSystem.displayBill();
break;
case 3:
billingSystem.saveToFile("items.txt");
cout << "Exiting program." << endl;
break;
default:
cout << "Invalid choice. Please try again." << endl;
}
} while (choice != 3);
return 0;
}
Output
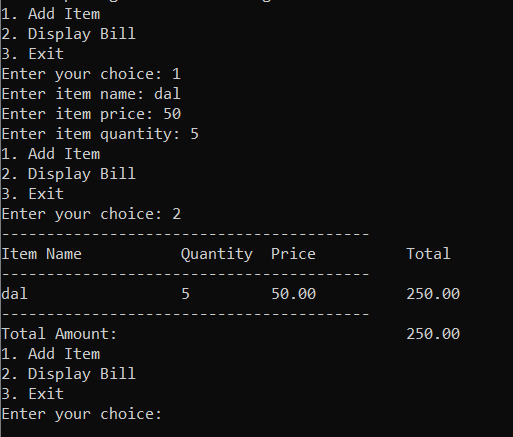
29. Write a class template that will have a single data member and other function members to add, subtract, multiply, and divide objects of that class template. Define your functions outside of your class and use the template class to create object using different data types.
Source Code:
#include <iostream>
using namespace std;
template <typename T>
class ArithmeticOperations {
private:
T value;
public:
ArithmeticOperations(T val) : value(val) {}
T getValue() {
return value;
}
void setValue(T val) {
value = val;
}
};
// Function to add two objects
template <typename T>
ArithmeticOperations<T> add(ArithmeticOperations<T> &obj1, ArithmeticOperations<T> &obj2) {
return ArithmeticOperations<T>(obj1.getValue() + obj2.getValue());
}
// Function to subtract two objects
template <typename T>
ArithmeticOperations<T> subtract(ArithmeticOperations<T> &obj1, ArithmeticOperations<T> &obj2) {
return ArithmeticOperations<T>(obj1.getValue() - obj2.getValue());
}
// Function to multiply two objects
template <typename T>
ArithmeticOperations<T> multiply(ArithmeticOperations<T> &obj1, ArithmeticOperations<T> &obj2) {
return ArithmeticOperations<T>(obj1.getValue() * obj2.getValue());
}
// Function to divide two objects
template <typename T>
ArithmeticOperations<T> divide(ArithmeticOperations<T> &obj1, ArithmeticOperations<T> &obj2) {
if (obj2.getValue() == 0) {
cerr << "Error: Division by zero!" << endl;
exit(1);
}
return ArithmeticOperations<T>(obj1.getValue() / obj2.getValue());
}
int main() {
// Create objects using different data types
ArithmeticOperations<int> intObj1(10);
ArithmeticOperations<int> intObj2(5);
ArithmeticOperations<double> doubleObj1(7.5);
ArithmeticOperations<double> doubleObj2(2.5);
// Perform operations and display results
cout << "Integer addition: " << add(intObj1, intObj2).getValue() << endl;
cout << "Double subtraction: " << subtract(doubleObj1, doubleObj2).getValue() << endl;
cout << "Integer multiplication: " << multiply(intObj1, intObj2).getValue() << endl;
cout << "Double division: " << divide(doubleObj1, doubleObj2).getValue() << endl;
return 0;
}
Output:
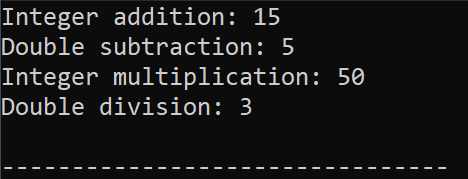
Do follow Notes IOE on Facebook and Instagram