lab 6 – OOP (Inheritance)
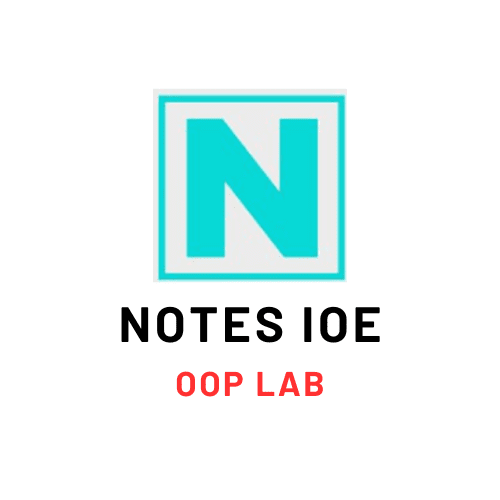
Learn the best practice of writing code in OOP using the concept of Inheritance and be an expert!
Theory
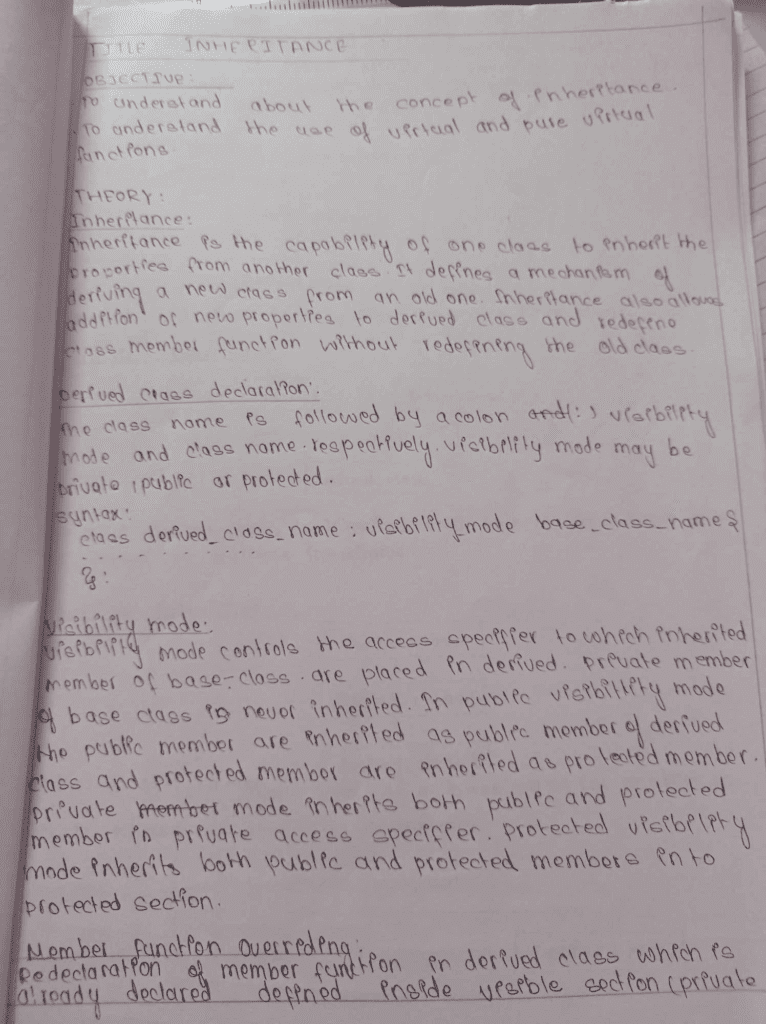
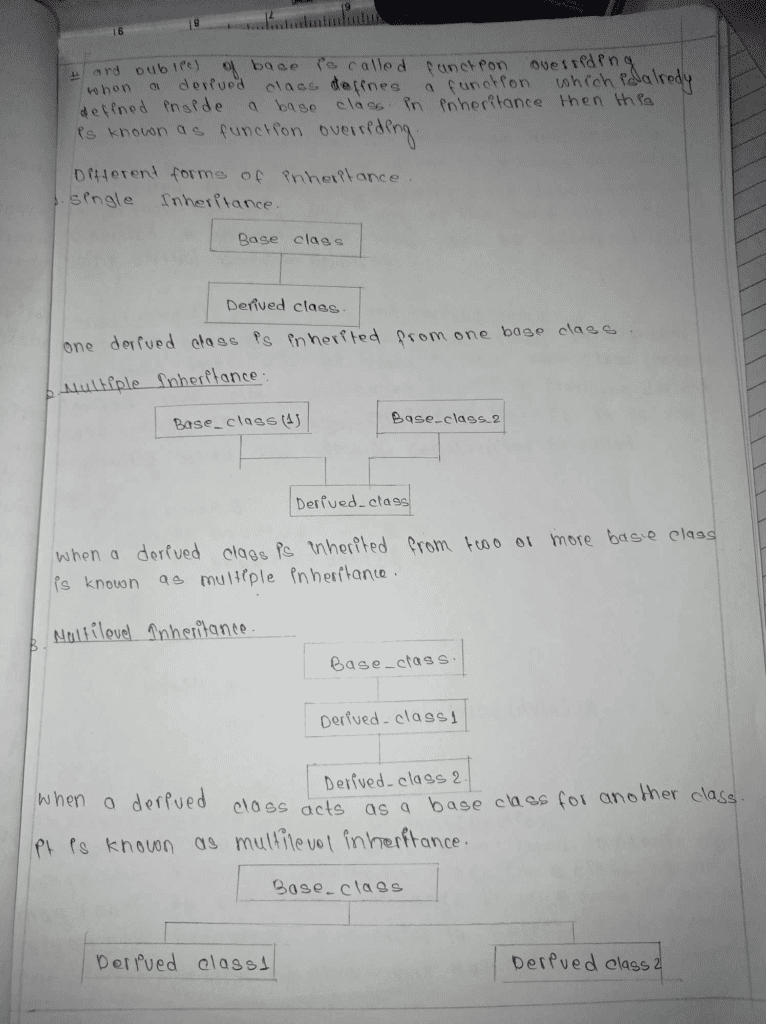
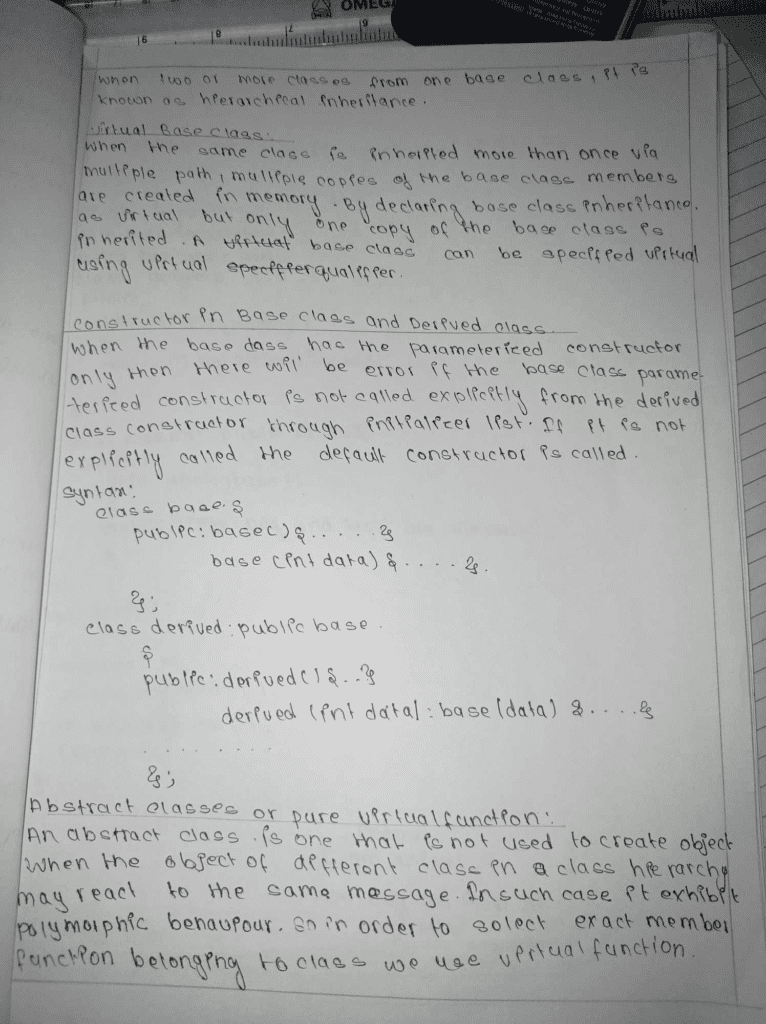
Minimize the Theory According to your wish!
1. Write a program to illustrate single, multiple, multilevel, hierarchical
inheritance.
Source Code:
#include <iostream>
using namespace std;
// Single Inheritance
class Animal {
public:
void eat() {
cout << "Animal is eating." << endl;
}
};
class Dog : public Animal {
public:
void bark() {
cout << "Dog is barking." << endl;
}
};
// Multiple Inheritance
class Flyable {
public:
void fly() {
cout << "Can fly." << endl;
}
};
class Bird : public Animal, public Flyable {
public:
void chirp() {
cout << "Bird is chirping." << endl;
}
};
// Multilevel Inheritance
class Mammal : public Animal {
public:
void giveBirth() {
cout << "Mammal is giving birth." << endl;
}
};
class Elephant : public Mammal {
public:
void trumpet() {
cout << "Elephant is trumpeting." << endl;
}
};
// Hierarchical Inheritance
class Cat : public Animal {
public:
void meow() {
cout << "Cat is meowing." << endl;
}
};
class Lion : public Cat {
public:
void roar() {
cout << "Lion is roaring." << endl;
}
};
int main() {
// Single Inheritance
Dog dog;
dog.eat();
dog.bark();
// Multiple Inheritance
Bird bird;
bird.eat();
bird.fly();
bird.chirp();
// Multilevel Inheritance
Elephant elephant;
elephant.eat();
elephant.giveBirth();
elephant.trumpet();
// Hierarchical Inheritance
Lion lion;
lion.eat();
lion.meow();
lion.roar();
return 0;
}
Output:
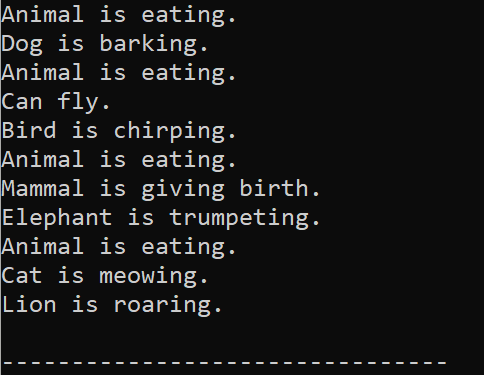
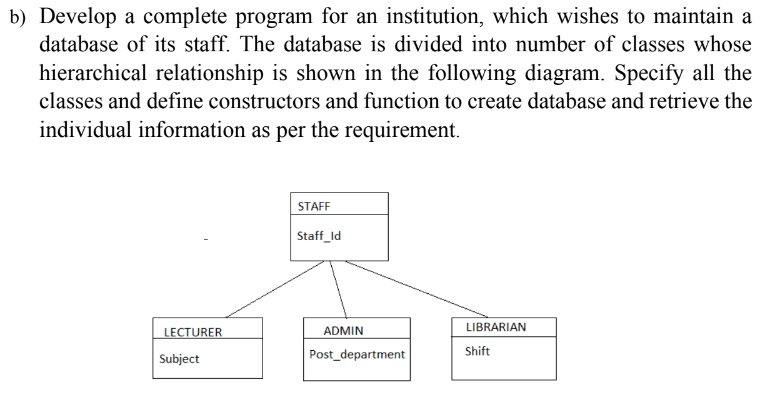
Source Code:
#include <iostream>
using namespace std;
//base class
class staff{
protected:
int id;
public:
staff (int a){
}
void getdata(){
cout<<"Enter Staff ID: ";
cin>>id;
}
void showdata(){
cout<<"Stadd ID: "<<id<<endl;
}
};
//derived class
class lecturer:public staff{
protected:
char sub[10];
public:
//calling staff constructor
lecturer(int a):staff(a) {}
void getdata(){
staff::getdata();//calling stadd getdata for id
cout<<"You are into lecturer class"<<endl;
cout<<"Enter subject: "<<endl;
cin>>sub;
}
void showdata(){
cout<<"The subject of lecturer is"<<sub<<endl;
}
};
//derived class second
class admin:public staff{
protected:
char post[10];
public:
admin(int a):staff(a){}
void getinfo(){
cout<<"You are into admin class"<<endl;
cout<<"Enter post in department: "<<endl;
cin>>post;
}
void showinfo(){
cout<<"Post in department is: "<<post<<endl;
}
};
//derived class third
class librarian:public staff{
protected:
char shift[10];
public:
librarian(int a):staff(a){}
void geti(){
staff::getdata();
cout<<"You are into librarian class"<<endl;
cout<<"Enter shift"<<endl;
cin>>shift;
}
void showi(){
cout<<"shift is"<<shift<<endl;
}
};
int main(){
lecturer l(1);
admin a(2);
librarian li(3);
l.getdata();
a.getinfo();
li.geti();
l.showdata();
a.showinfo();
li.showi();
return 0;
}
Output:
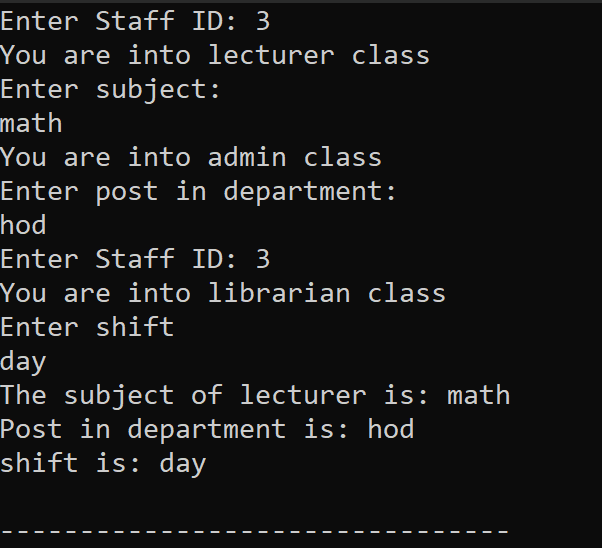
3. Write a program to find the area of a rectangle by passing length and breadth
as arguments after creating member functions in both derived and base class
with the same name.
Source Code:
#include <iostream>
using namespace std;
class Shape {
protected:
double area;
public:
//Default constructor
Shape() : area(0.0) {}
void calculateArea(double length, double breadth) {
area = length * breadth;
}
void displayArea() {
cout << "Area: " << area << endl;
}
};
//derived class
class Rectangle : public Shape {
public:
void calculateArea(double length, double breadth) {
area = length * breadth;
}
};
int main() {
double length, breadth;
cout << "Enter length: ";
cin >> length;
cout << "Enter breadth: ";
cin >> breadth;
Shape shape;
shape.calculateArea(length, breadth);
cout << "Using Base Class: ";
shape.displayArea();
Rectangle rectangle;
rectangle.calculateArea(length, breadth);
cout << "Using Derived Class: ";
rectangle.displayArea();
return 0;
}
Output:
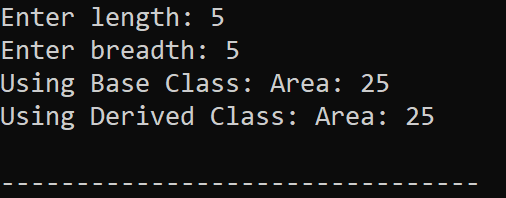
4. Write a program to show the use of virtual base class and pure virtual
function.
Source Code:
#include <iostream>
using namespace std;
// Virtual Base Class
class Shape {
public:
virtual double calculateArea() = 0; // Pure virtual function
virtual void display() {
cout << "Shape" << endl;
}
};
// Derived Classes
class Circle : public Shape {
private:
double radius;
public:
Circle(double r) : radius(r) {}
double calculateArea() {
return 3.14159 * radius * radius;
}
void display() {
cout << "Circle" << endl;
}
};
class Rectangle : public Shape {
private:
double length;
double width;
public:
Rectangle(double l, double w) : length(l), width(w) {}
double calculateArea() {
return length * width;
}
void display() {
cout << "Rectangle" << endl;
}
};
int main() {
Circle circle(5.0);
Rectangle rectangle(4.0, 6.0);
Shape* shape1 = &circle;
Shape* shape2 = &rectangle;
shape1->display();
cout << "Area: " << shape1->calculateArea() << endl;
shape2->display();
cout << "Area: " << shape2->calculateArea() << endl;
return 0;
}
Output:
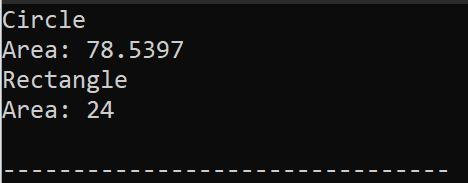
Find More Content on Notes IOE, Happy Learning!!
Do follow Us on Facebook and Instagram