lab 4,5 – OOP (Operator Overloading)
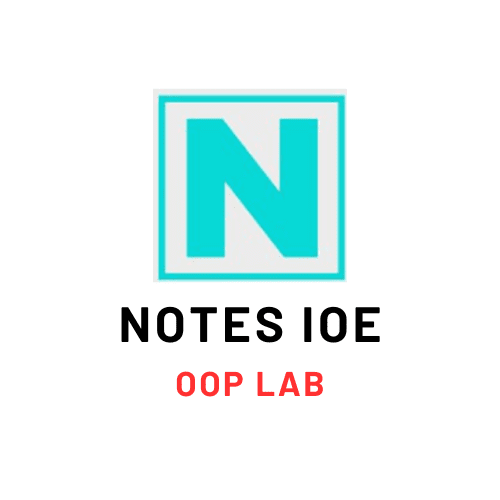
LAB – 4
Theory
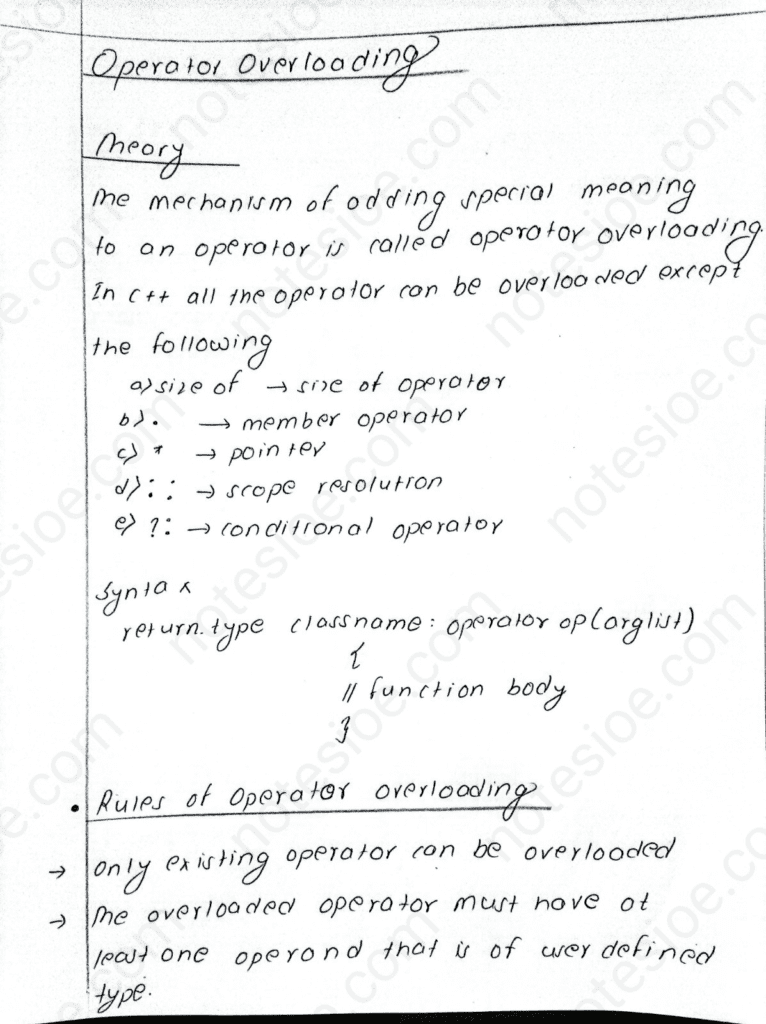
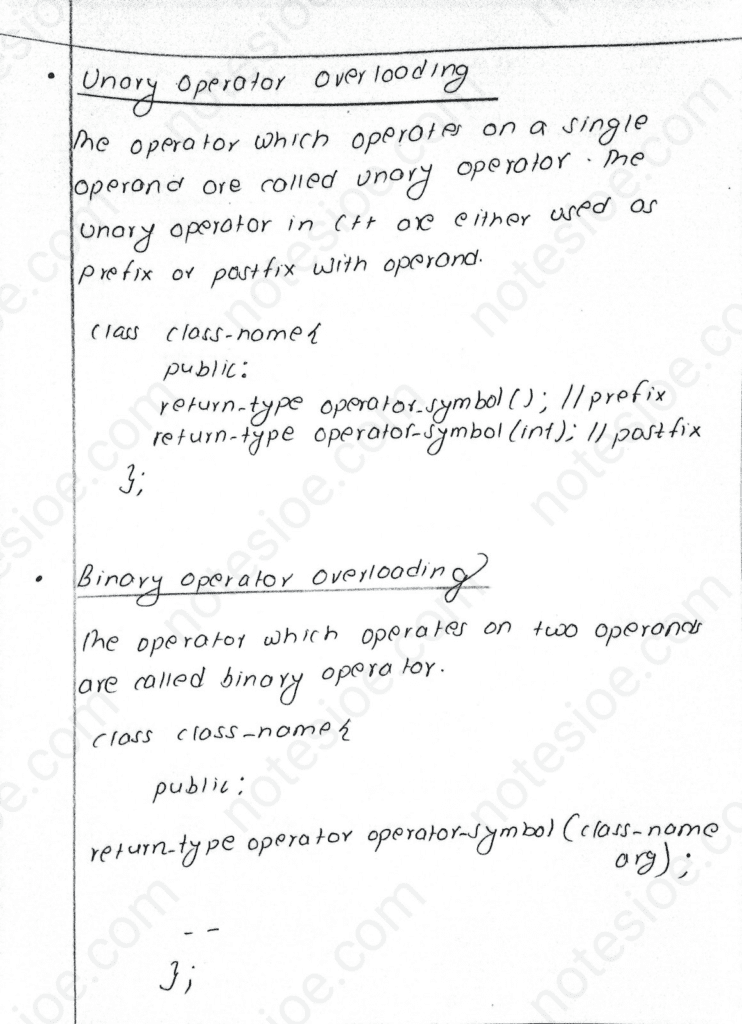
1. WAP to show operator function returns object of type complex
#include <iostream>
using namespace std;
class complex {
private:
float real, img;
public:
complex() {
real = 0.0;
img = 0.0;
}
complex(float rl, float im) {
real = rl;
img = im;
}
void display() {
cout << real << "," << img;
}
complex operator +(complex c);
};
complex complex :: operator+(complex c){
complex temp;
temp.real = real + c.real;
temp.img = img + c.img;
return temp;
}
int main() {
complex c1, c2, c3;
c1 = complex(4.4, 4.4);
c2 = complex(3.3, 3.3);
c3 = c1 + c2;
c3.display();
return 0;
}
2. WAP to overload an increment operator (++) in prefix notation.
#include <iostream>
using namespace std;
class counter{
private:
int count;
public:
counter(){ //constructor
count=0;
}
int ret_count(){
return count;
}
void operator ++(){
count++; //increment
}
};
int main(){
counter c1,c2;
cout<<"\nc1="<<c1.ret_count(); //calling ret_count function which will return 0 initially
cout<<"\nc2="<<c2.ret_count(); //calling ret_count function which will return 0 initially
++c1; //initially 0 now value will be 1
++c2; //initially 0 now value will be 1
++c2; //value was 1 no it changes to 2;
cout<<"\nc1="<<c1.ret_count(); //printing value
cout<<"\nc2="<<c2.ret_count(); //priniting value
return 0;
}
3. WAP to overload unary minus operator using friend function.
#include <iostream>
using namespace std;
class counter {
private:
int count;
public:
counter() {
count = 0;
}
void getdata() {
cout << "Enter a number: ";
cin >> count;
}
int ret_count() {
return count;
}
friend counter operator--(counter& c, int);
};
counter operator--(counter& c, int) {
counter temp;
temp.count = c.count--;
return temp;
}
int main() {
counter c1;
cout << "\nc1=";
c1.getdata();
cout << "c1=" << c1.ret_count();
c1--;
cout << "\nc1=" << c1.ret_count();
return 0;
}
4. WAP to overload plus operator to add two complex number using friend
function and without using friend function.
A) Using Friend Function
#include <iostream>
using namespace std;
class complex {
private:
float real, img;
public:
complex() {
real = 0.0;
img = 0.0;
}
complex(float rl, float im) {
real = rl;
img = im;
}
void display() {
cout << real << "," << img;
}
friend complex operator+(complex c1, complex c2);
};
complex operator+(complex c1, complex c2) {
complex temp;
temp.real = c1.real + c2.real;
temp.img = c1.img + c2.img;
return temp;
}
int main() {
complex c1, c2, c3;
c1 = complex(4.4, 4.4);
c2 = complex(3.3, 3.3);
c3 = c1 + c2;
c3.display();
return 0;
}
B) Without Using Friend Function
#include <iostream>
using namespace std;
class complex {
private:
float real, img;
public:
complex() {
real = 0.0;
img = 0.0;
}
complex(float rl, float im) {
real = rl;
img = im;
}
void display() {
cout << real << "," << img;
}
complex operator +(complex c);
};
complex complex :: operator+(complex c){
complex temp;
temp.real = real + c.real;
temp.img = img + c.img;
return temp;
}
int main() {
complex c1, c2, c3;
c1 = complex(4.4, 4.4);
c2 = complex(3.3, 3.3);
c3 = c1 + c2;
c3.display();
return 0;
}
LAB – 5
1. WAP to convert hour into object of a class Time which has minute and second as members.
#include <iostream>
using namespace std;
class time{
private:
int minute, second;
public:
time(){
second=0;
minute=0;
}
time(float c){
second = c * 60 *60;
minute = c *60;
}
void display(){
cout<<"Minute = "<<minute;
cout<<"\nSecond = "<<second;
}
};
int main(){
time t;
float h;
cout<<"Enter Hour: ";
cin>>h;
t=h;
t.display();
return 0;
}
2. WAP to convert Time expressed in minute and second into single value hour.
#include <iostream>
using namespace std;
class time{
private:
int minute,second;
public:
time(){
minute=0;
second=0;
}
void getdata(){
cout<<"Enter Minute: ";
cin>>minute;
cout<<"Enter Second: ";
cin>>second;
}
operator float(){
float hour = ((minute/60) + (second/3600));
return hour;
}
};
int main(){
time t;t.getdata();
float h;
h=t;
cout<<"Hour= "<<h;
return 0;
}
c) Create a class named Centigrade and another class name Fahrenheit. Write a program to convert object of Centigrade class into object of another class Fahrenheit.
i. Define the conversion routine in source class.
ii. Define the conversion routine in destination class
(For centigrade to Fahrenheit conversion, multiply by 9, then divide by 5, thenadd 32).
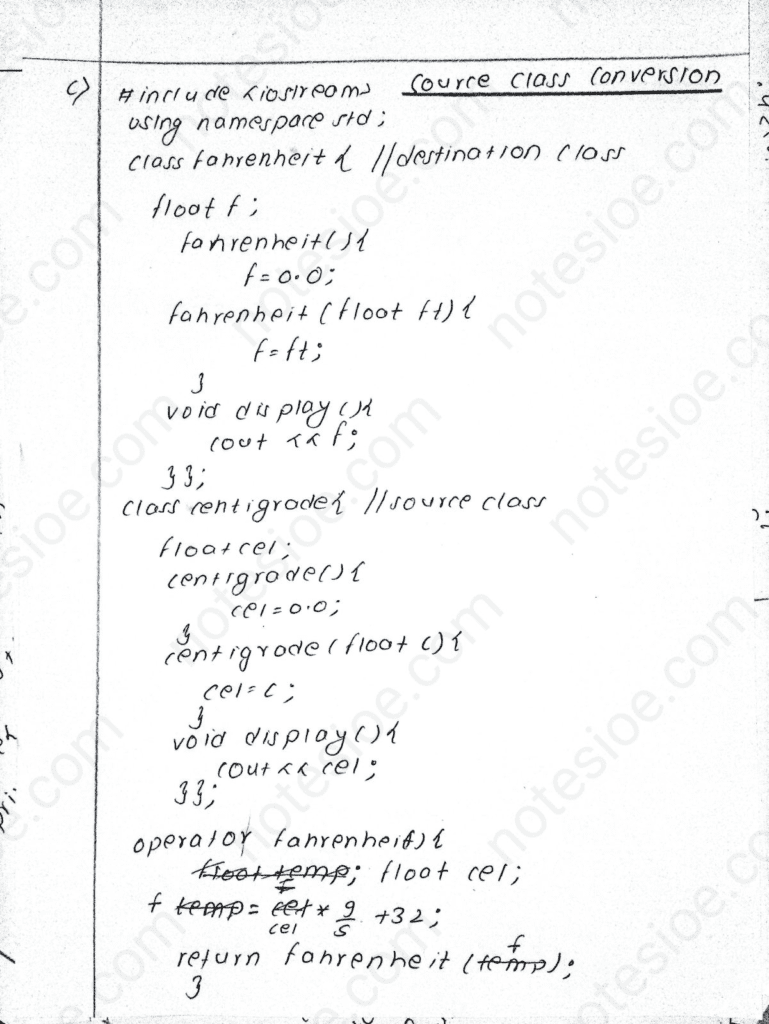
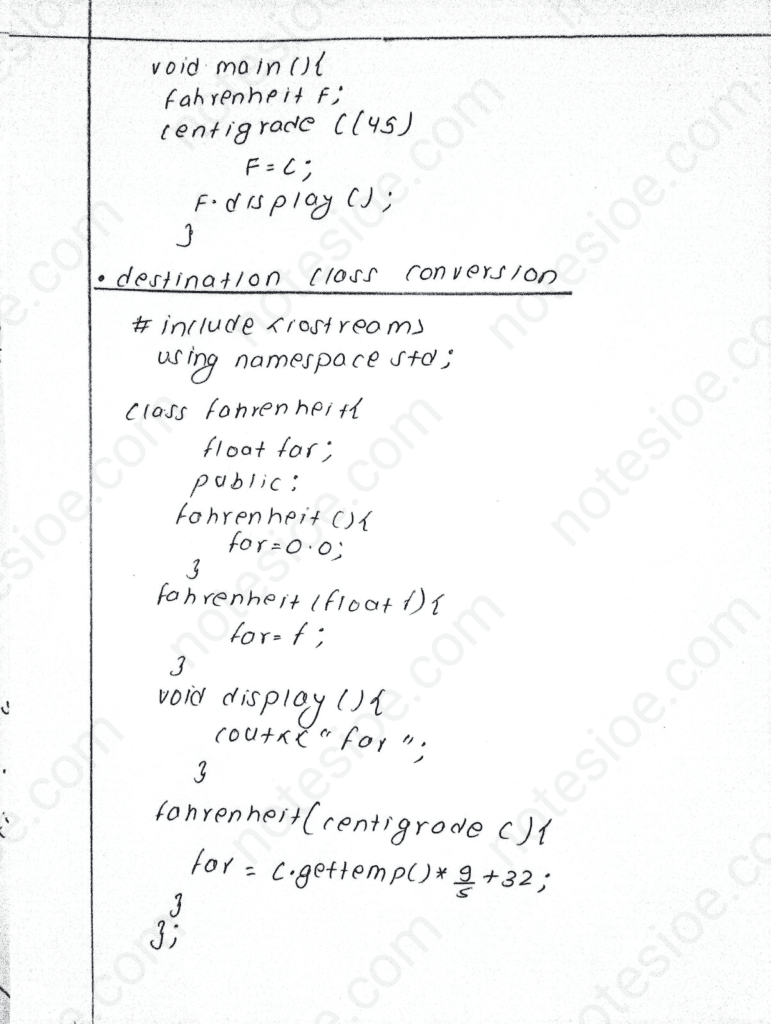
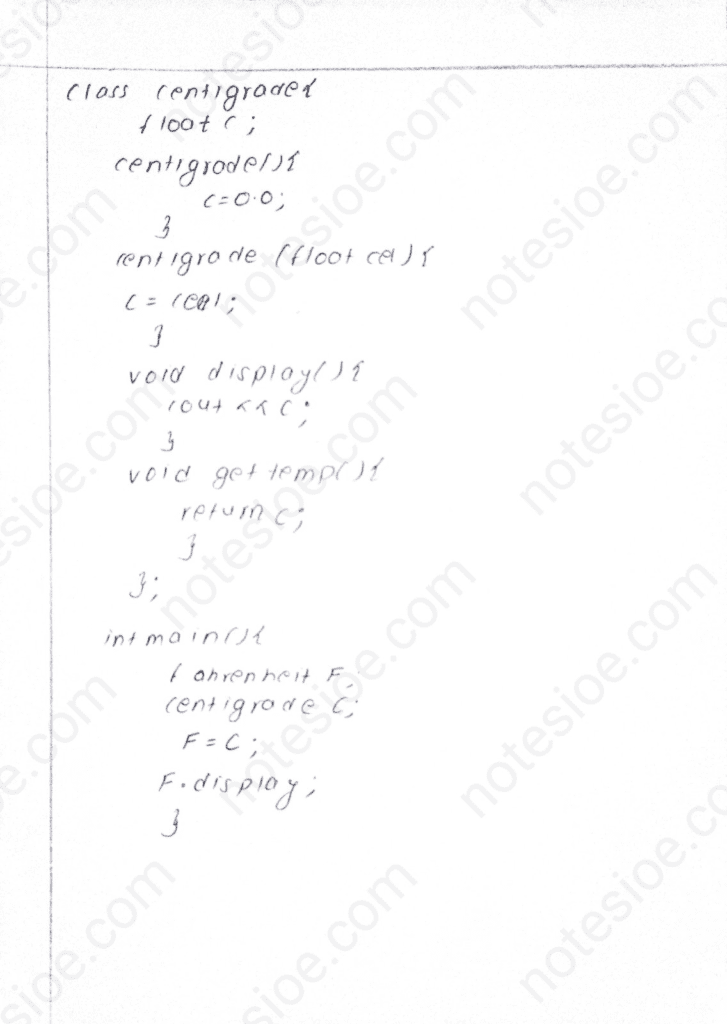
Notes IOE – Taking Learning Seriously But Not Ourselves!
Do follow our Facebook and Instagram