Lab 3 – OOP (Constructor)
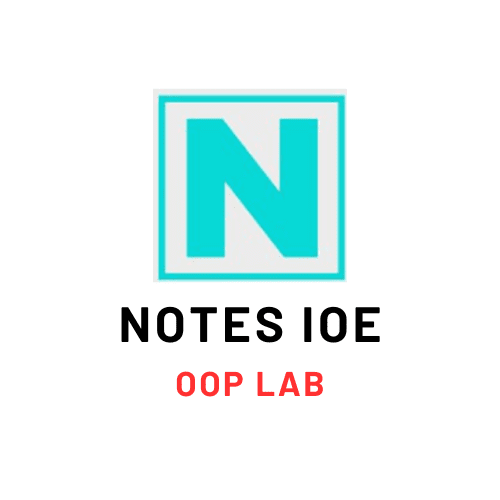
Theory for Constructor
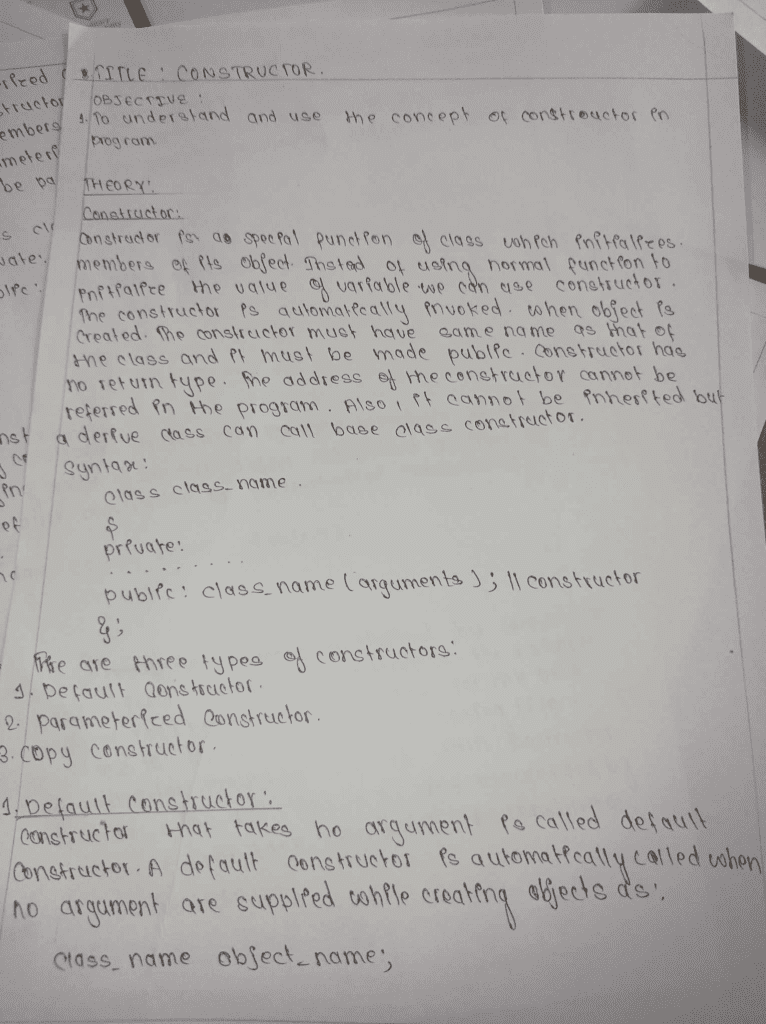
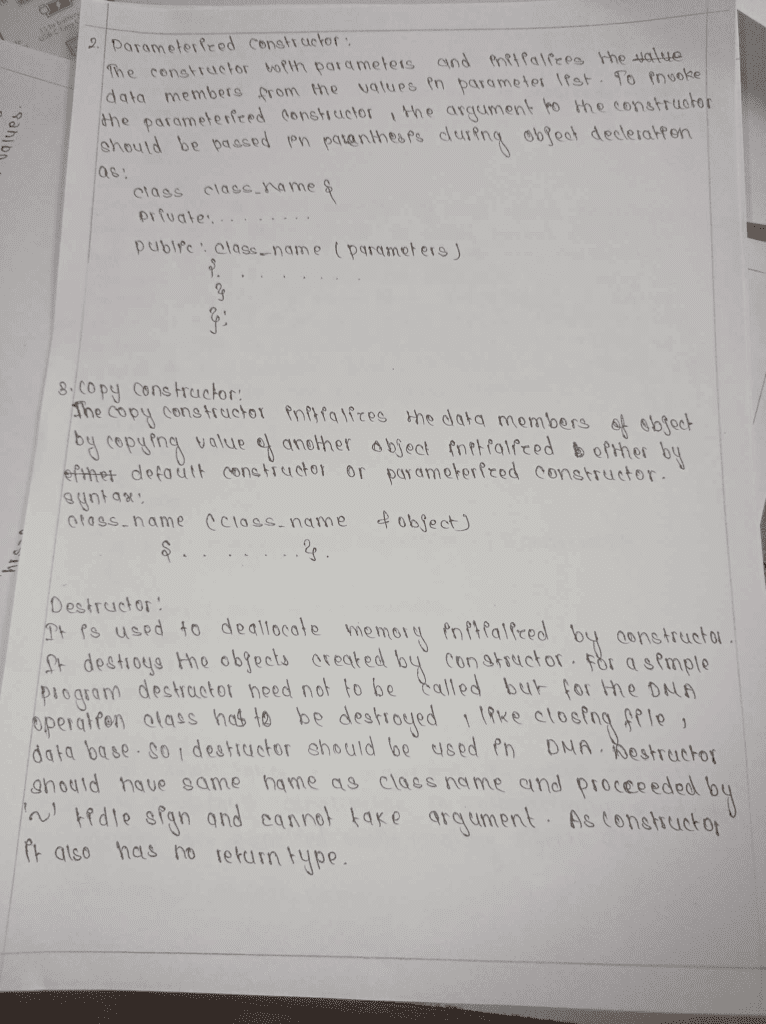
Codes for Constructor
Write a program that displays the total time in 24-hour clock format having
fixed time 12:34:56 and 10:35:14. One constructor should initialize member
data hour, minute, and second to 0 and another should initialize it to fixed
values. The other two member functions should add two objects of type time
passed as arguments and display the result.
#include <iostream>
using namespace std;
class time{
private:
int hour1,min1,sec1,hour2,min2,sec2;
public:
time(){
hour1=0;
min1=0;
sec1=0;
hour2=0;
min2=0;
sec2=0;
}
time(int h1,int m1, int s1,int h2,int m2,int s2){
hour1=h1;
min1=m1;
sec1=s1;
hour2=h2;
min2=m2;
sec2=s2;
}
int add(){
int t1,t2,t3,day=0;
cout<<"The sum is: "<<endl;
t1=hour1+hour2;
t2=min1+min2;
t3=sec1+sec2;
if(t1>=24){
t1=t1-24;
day++;
}
if(t2>=60){
t2=t2-60;
t1++;
}
if(t3>=60){
t3=t3-60;
t2++;
}
cout<<"HOUR= "<<t1;
cout<<"MIN= "<<t2;
cout<<"SEC= "<<t3;
}
};
int main(){
time t(12,34,56,10,35,14);
t.add();
}
WAP to perform the addition of distance in the feet and inches format. Use
objects as argument.
#include <iostream
using namespace std;
class Distance{
private:
int feet;
int inch;
public:
Distance(){//default constructor
feet = 0;
inch = 0;
}
void getDistance(){
cout<<"Enter feet: ";
cin>>feet;
cout<<"Enter inches: ";
cin>>inch;
}
void showDistance(){
cout<<"Feet: "<<feet<<endl<<"Inches: "<<inch<<endl;
}
Distance addDistance(Distance d1, Distance d2){//takes object of distance as argument and returns distance as object
Distance sum;
sum.feet = d1.feet+d2.feet;
sum.inch = d1.inch+d2.inch;
if(sum.inch>=12){//condition
sum.inch=sum.inch-12;
sum.feet++;
}
}
};
int main() {
Distance d1, d2, d3;
cout<<"Enter the first distance:"<< endl;
d1.getDistance();
cout<<"Enter the second distance:"<< endl;
d2.getDistance();
d3 = d1.addDistance(d1,d2);
cout << "Sum of distances: ";
d3.showDistance();
return 0;
}
Create a class first with data member data1 and another class second with data
member data2. Display the largest number. Use friend function.
#include <iostream>
using namespace std;
class second; // Forward declaration of the second class
class first {
int data1;
public:
void getdata1() {
cout << "Enter First Data: ";
cin >> data1;
}
friend int larger(first, second);
};
class second {
int data2;
public:
void getdata2() {
cout << "Enter Second Data: ";
cin >> data2;
}
friend int larger(first, second);
};
int larger(first a, second b) {
if (a.data1 > b.data2) {
return a.data1;
} else {
return b.data2;
}
}
int main() {
first a;
second b;
a.getdata1();
b.getdata2();
cout << "\nThe Larger Value Is: " << larger(a, b);
return 0;
}
Write a simple program that convert the temperature in degree centigrade to degree Fahrenheit and vice-versa using the basic concept of classes and objects. Make separate class for centigrade and Fahrenheit which have the private member to hold the values and add conversion function in each class from centigrade to Fahrenheit.
#include <iostream>
using namespace std;
class Celsius {
float c;
public:
Celsius() {
c = 0.0;
}
Celsius(float a) {
c = a;
}
void display() {
cout << "The temperature in Celsius is: " << c;
}
};
class Fahrenheit {
float f;
public:
Fahrenheit() {
f = 0.0;
}
void display() {
cout << "The temperature in Fahrenheit is: " << f;
}
Fahrenheit(float fTemp) {
f = ((fTemp * 9 / 5) + 32);
}
};
int main() {
Fahrenheit far;
float cel;
cout << "Enter temperature in Celsius: ";
cin >> cel;
far = cel;
far.display();
return 0;
}
——Notes IOE——
Do follow our Facebook and Instagram