Lab 2 – OOP (Functions in C++)
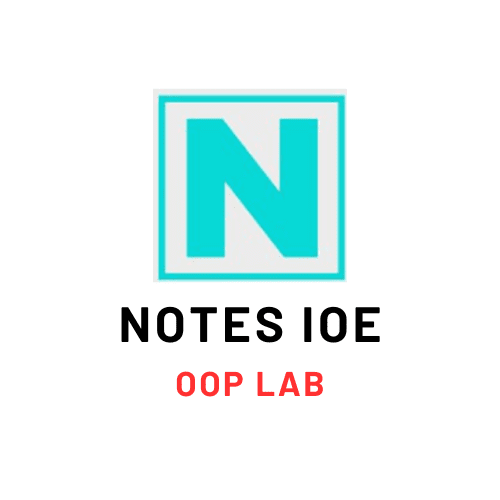
Theory
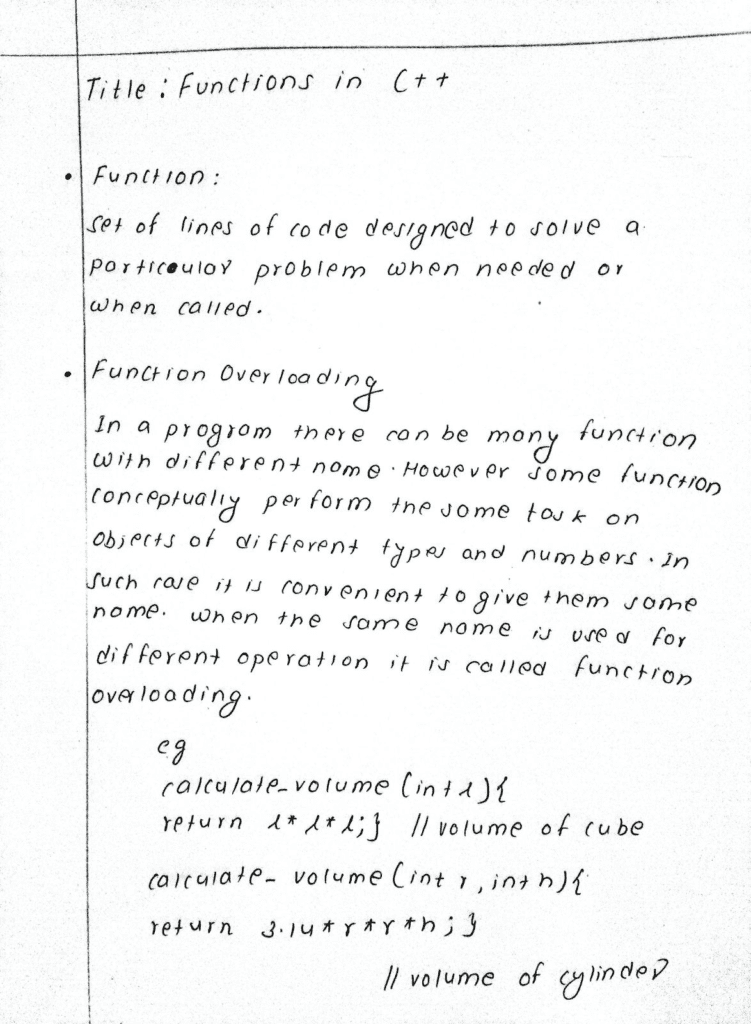
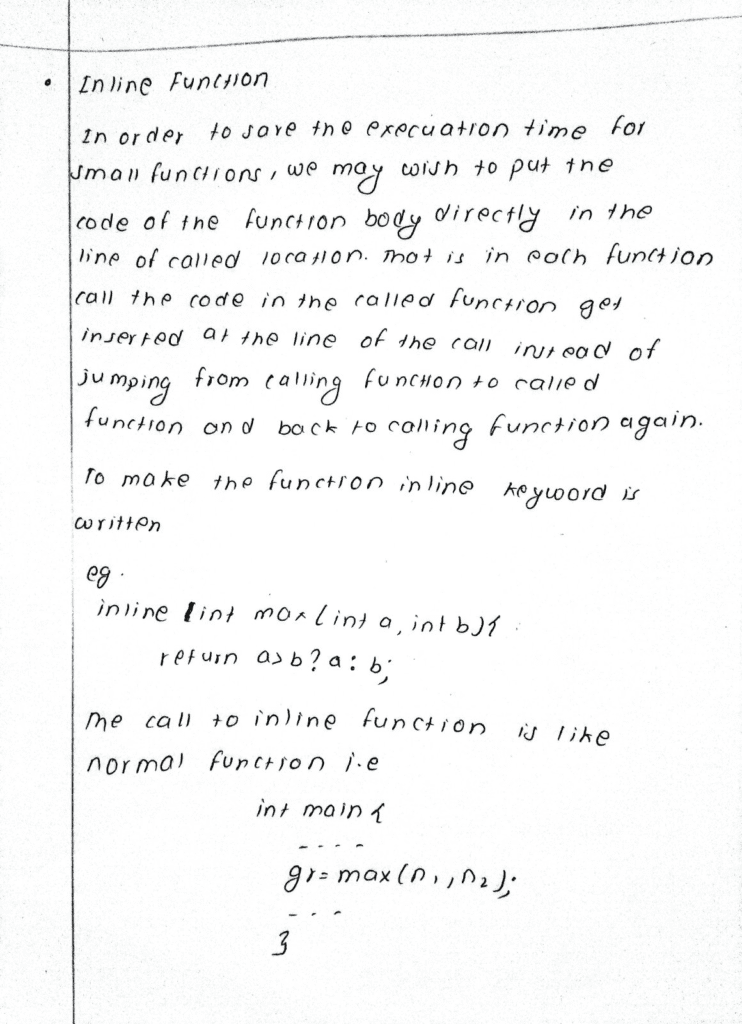
Write a program to find the volume of cube, cylinder and rectangular box by using the concept of function of overloading.
Code
#include <iostream>
#include <cmath>
#define PI 3.14
using namespace std;
class Volume {
public:
float calculateVolume(float sidelength) {
return pow(sidelength, 3);
}
float calculateVolume(float radius, float height) {
return PI * pow(radius, 2) * height;
}
float calculateVolume(float length, float breadth, float rectangularHeight) {
return length * breadth * rectangularHeight;
}
};
//ACE078BCT071
int main() {
Volume v;
float sidelength, length, breadth, cylinderHeight, radius, rectangularHeight;
// Cube
cout << "Enter the side length of the cube: ";
cin >> sidelength;
// Cylinder
cout << "Enter the radius of the cylinder: ";
cin >> radius;
cout << "Enter the height of the cylinder: ";
cin >> cylinderHeight;
// Rectangular box
cout << "Enter the length of the rectangular box: ";
cin >> length;
cout << "Enter the width of the rectangular box: ";
cin >> breadth;
cout << "Enter the height of the rectangular box: ";
cin >> rectangularHeight;
cout << "\nVolume of the cube is: " << v.calculateVolume(sidelength);
cout << "\nVolume of the cylinder is: " << v.calculateVolume(radius, cylinderHeight);
cout << "\nVolume of the rectangle is: " << v.calculateVolume(length, breadth, rectangularHeight);
return 0;
}
Image
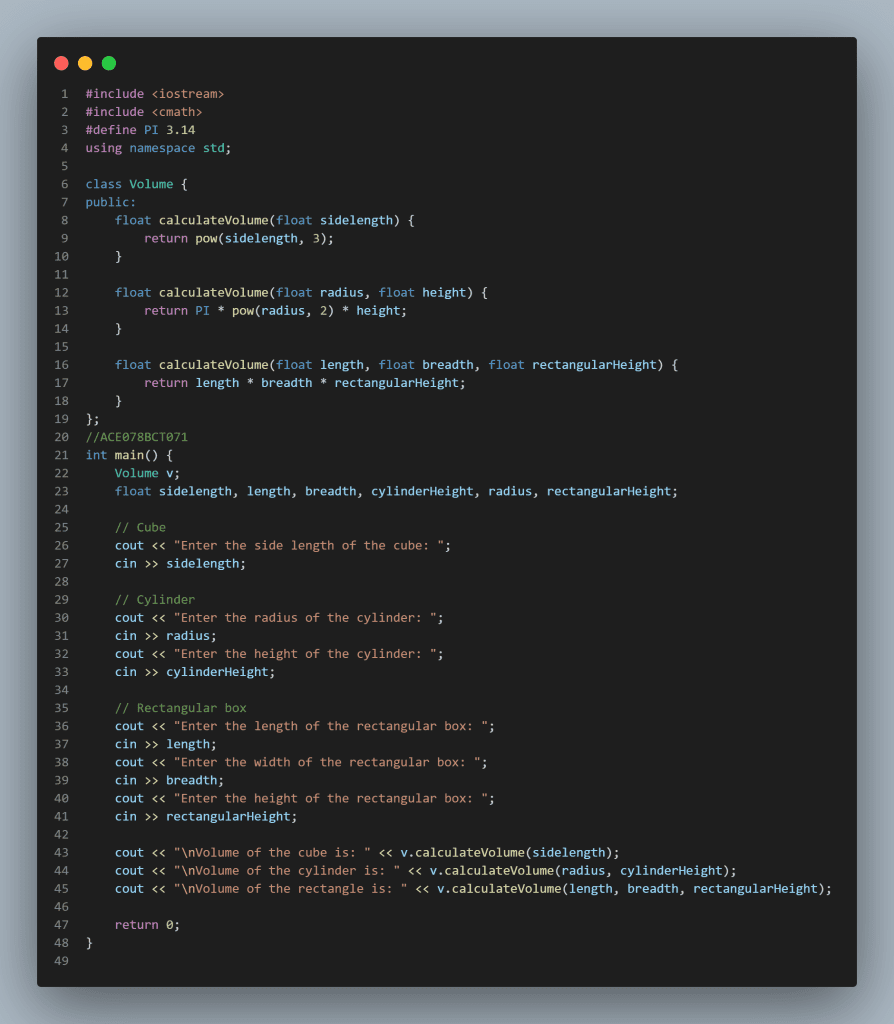
Output
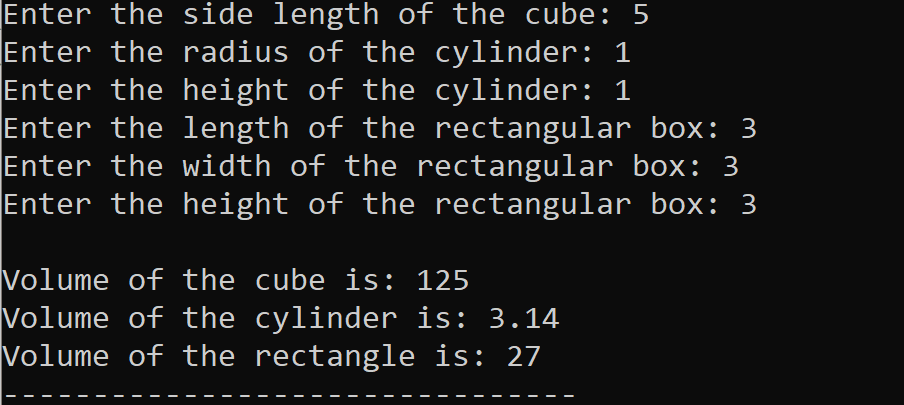
Write a program to display n characters by using default arguments for all
parameters. Assume that the function takes two arguments (one character to be
printed and other number of characters to be printed).
Code
#include <iostream>
using namespace std;
class loop{
public:
void looping(int n=5,char a= 's'){//default arguments
int i;
for(i=1;i<=n;i++){
cout<<a<<endl;
}
}
//ACE078BCT071
};
int main(){
loop l; //making object of class loop
l.looping();
return 0;
}
Images
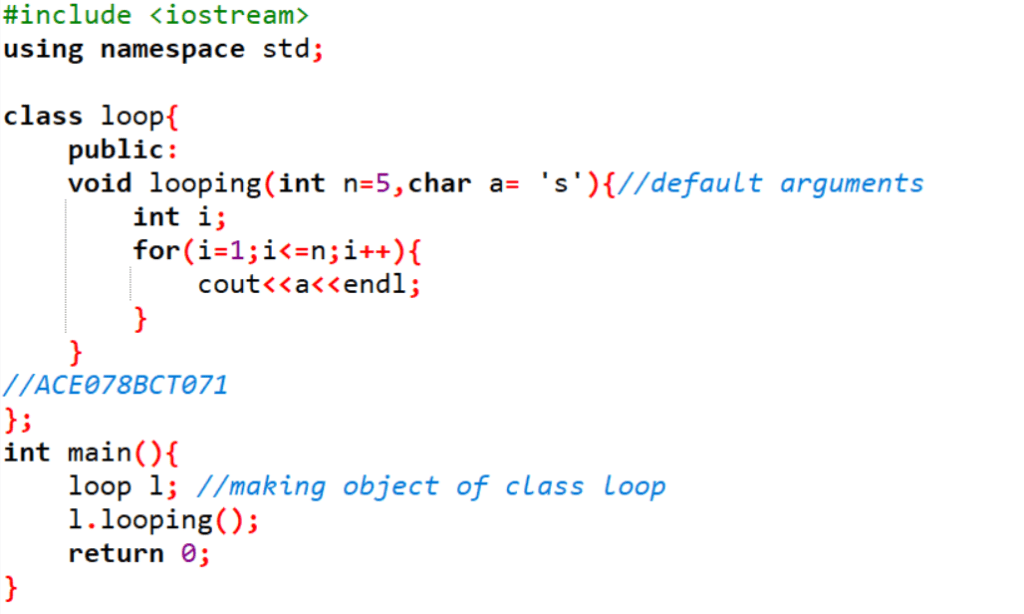
Output
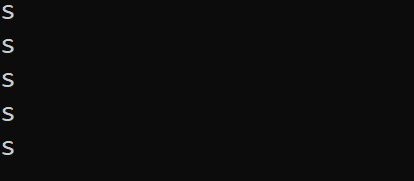
Assume that employee will have to pay 10 percent income tax to the
government. Ask user to enter the employee salary. Use inline function to
display the net payment to the employee by the company.
Code
#include <iostream>
using namespace std;
class employee{
private:
int salary;
public:
void getdata();//Defining function only
void vat();
};
inline void employee:: getdata(){//accesing function using inline
cout<<"Enter Salary: ";
cin>>salary;
}//ACE078BCT071
inline void employee::vat(){
float netsalary;
netsalary = salary - 0.1 * salary;
cout<<"\nThe salary after VAT is: "<<netsalary;
}
int main(){
employee e;//object creation
e.getdata();
e.vat();
return 0;
}
Images
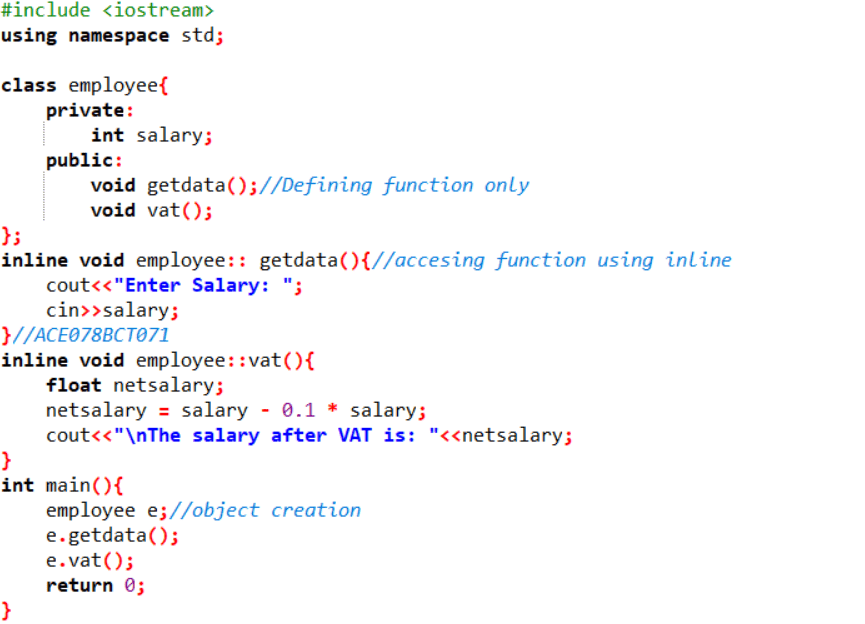
Output
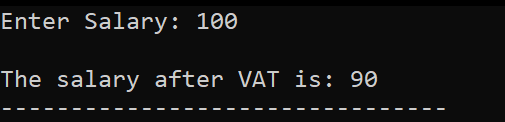
Write a function that passes two temperatures by reference and sets the larger
of the two numbers to 100 by using return by reference.
Code
#include <iostream>
using namespace std;
class compare_temp{
public:
int &temperature(int &a,int &b){//returning by reference
if(a>b){
return a;
}else{
return b;
}
}
};//ACE078BCT071
int main(){
compare_temp c;
int low_temp,high_temp;
//taking input
cout<<"Enter Higher temperature: ";
cin>>high_temp;
cout<<"\nEmter Lower Temperature: ";
cin>>low_temp;
cout<<"\nInitial Temperatures are: "<<high_temp<<" and "<<low_temp;
c.temperature(high_temp,low_temp)=100;//changing grater value
cout<<"\nFinal Temperatures are: "<<high_temp<<" and "<<low_temp;
return 0;
}
Images
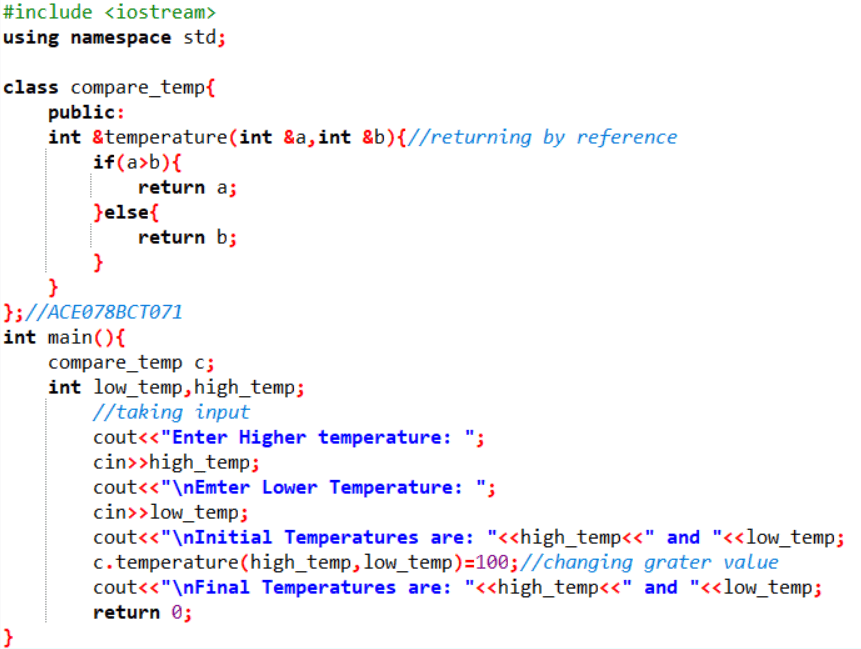
Output
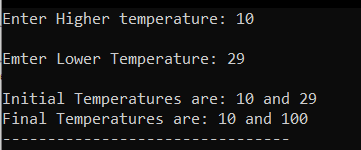
WAP to find the sum and average of the number by using new and delete
operator. Also use static_cast casting operator.
Code
#include<iostream>
using namespace std;
class sum{
private:
int n,i;
public:
void getdata(){
cout<<"How many numbers? ";
cin>>n;
}
void avg(){
float avg; int *p,tot=0;
p=new int[n];
for(i=0;i<n;i++){
cin>>p[i];
tot =tot+p[i];
}
avg = static_cast<float>(tot)/n;
cout<<"Total= "<<tot;
cout<<"Avg = "<<avg;
delete []p;
}
};
int main(){
sum s;
s.getdata();
s.avg();
return 0;
}
Notes IOE – Taking Learning Seriously But Not Ourselves
Do follow us on Facebook and Instagram