Lab 1 – OOP (Objects and Classes)
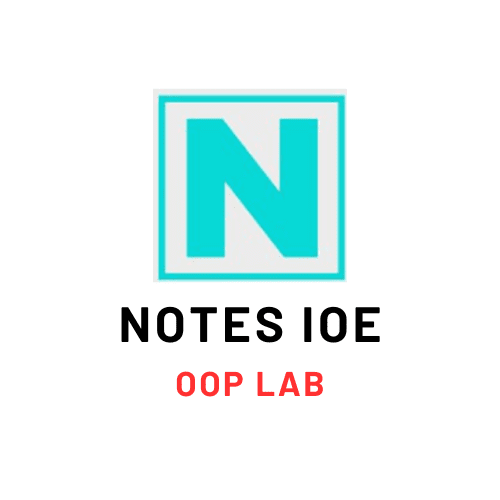
Theory
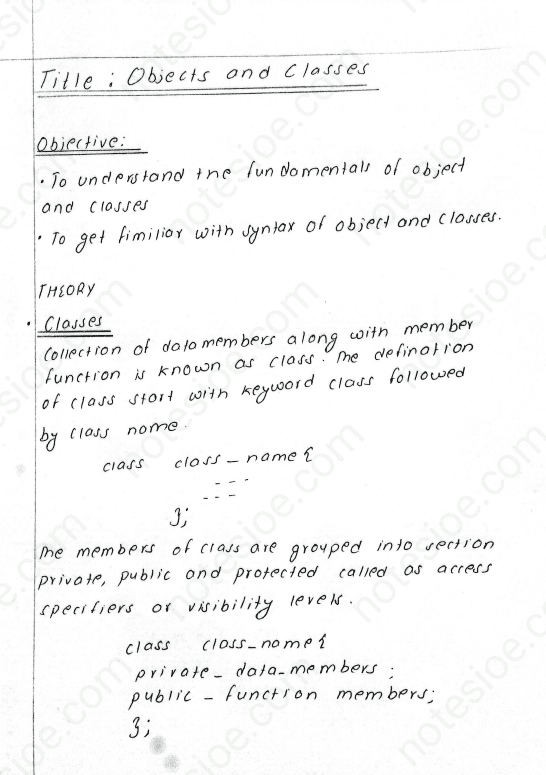
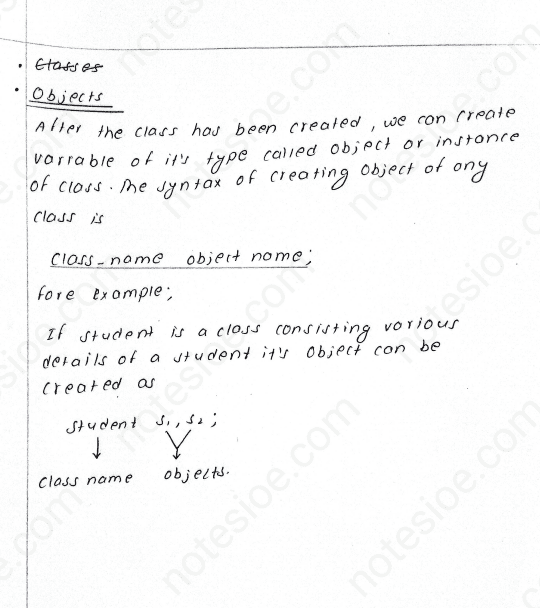
Program to Calculate Perimeter/Area of Rectangle
Code
#include<iostream>
using namespace std;
class rectangle{
public:
int perimeter(int l,int b){
return 2*(l+b);
}
public:
int area(int l,int b){
return l*b;
}
};
//Sohan (ACE078BCT071)
int main(){
rectangle r1;
int l,b;
cout<<"Enter Length of rectangle: ";
cin>>l;
cout<<"Enter Breadth of rectangle: ";
cin>>b;
cout<<"The perimeter of rectange is: "<<r1.perimeter(l,b);
cout<<"\nThe area of rectange is: "<<r1.area(l,b);
return 0;
}
Images
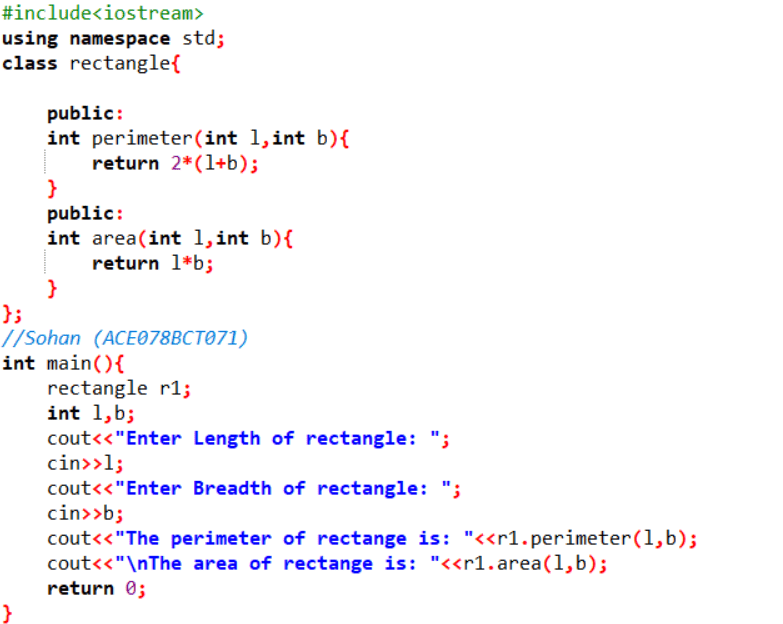
Output
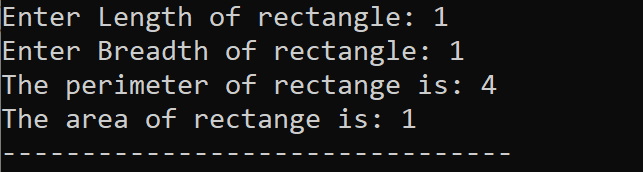
Program to Calculate Distance between two points
Code
#include<iostream>
#include <math.h>
using namespace std;
class length{
private:
int x1,x2,y1,y2;
public:
void input(){
cout<<"Enter X coordinates";
cout<<"\nEnter X1: ";
cin>>x1;
cout<<"\nEnter X2: ";
cin>>x2;
cout<<"Enter Y coordinates";
cout<<"\nEnter Y1: ";
cin>>y1;
cout<<"\nEnter Y2: ";
cin>>y2;
}
void dist(){
float d;
d = sqrt(pow((x2-x1),2)+pow((y2-y1),2));
cout<<"The Distance between two points is: "<<d<<"Units";
}
};
//Sohan (ACE078BCT071)
int main(){
length d1;
d1.input();
d1.dist();
return 0;
}
Images
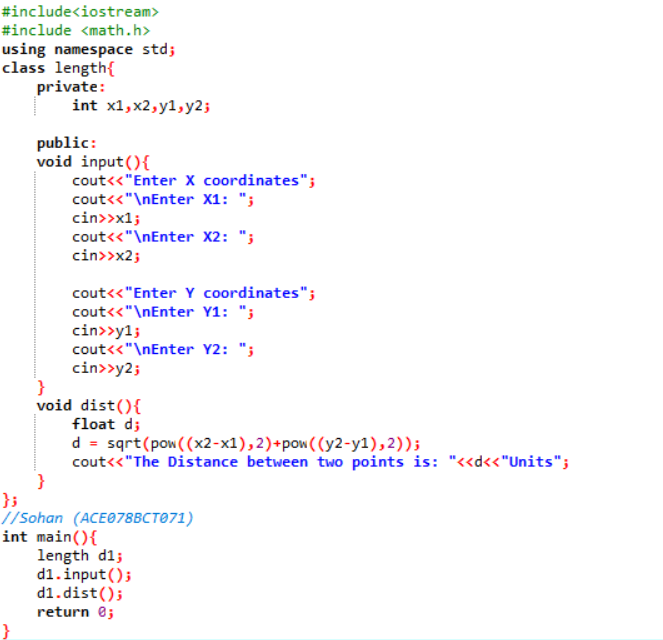
Output
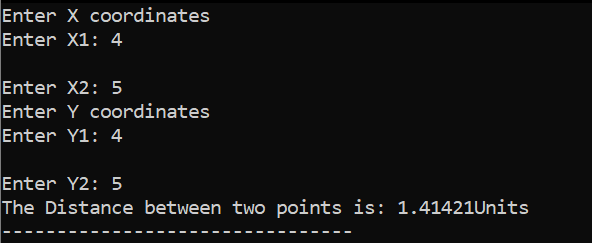
Program to calculate largest between to numbers largest number
Code
#include
using namespace std;
class largest{
private:
int a1,a2;
public:
void input(){
cout<<"Enter first number: ";
cin>>a1;
cout<<"\nEnter second number: : ";
cin>>a2;
}
void greatest(){
if(a1>a2){
cout<<a1<<" is greatest";
}else if(a1==a2){
cout<<"Both are equal";
}else{
cout<<a2<<" is greatest";
}
}
};
//Sohan (ACE078BCT071)
int main(){
largest l1;
l1.input();
l1.greatest();
return 0;
}
Images
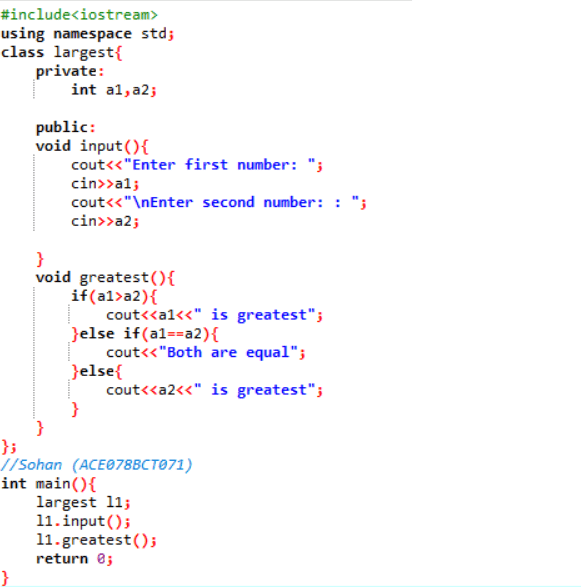
Output
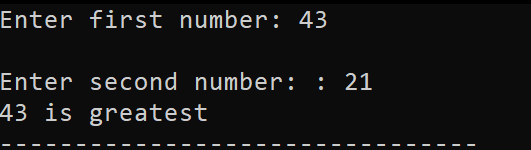
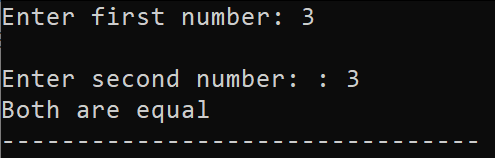
Notes IOE – Taking Learning Seriously But Not Ourselves!
Do follow our Facebook and Instagram